Power Apps Text Functions (With Examples)
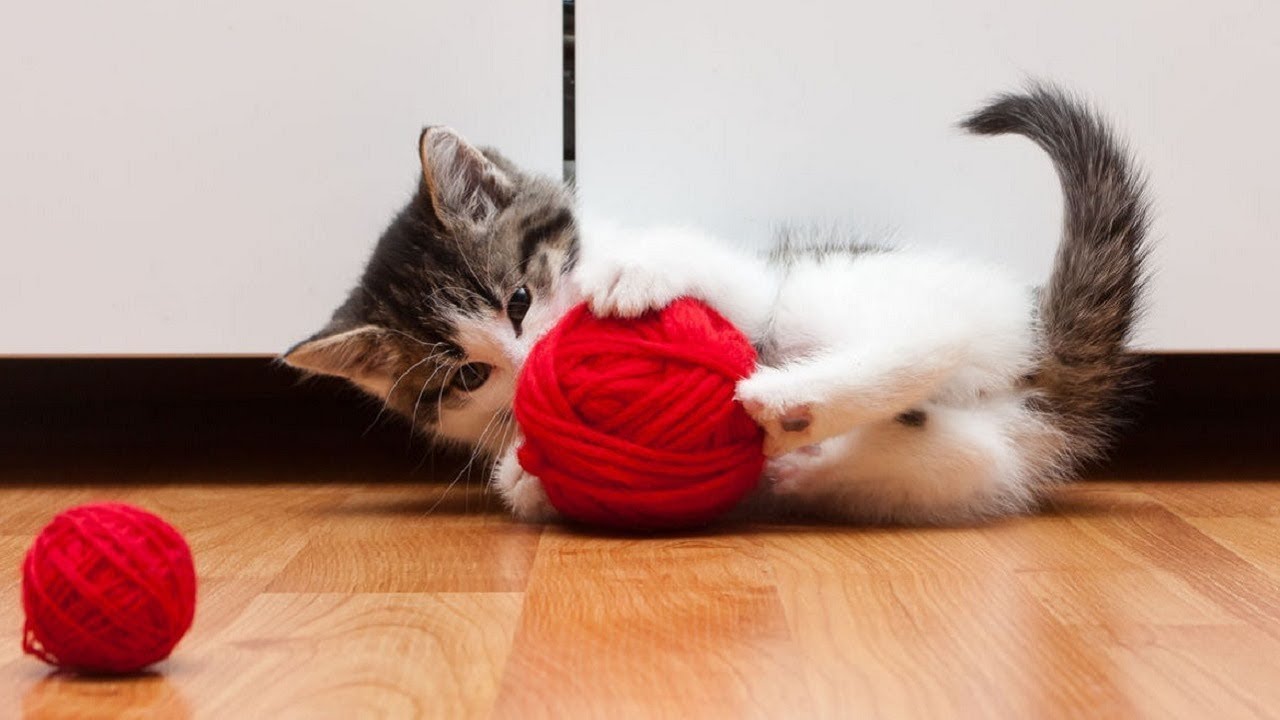
Text is the most common data type in Power Apps. Often, it needs to be manipulated in some way: extract part of the text, format it differently or remove unnecessary spaces or symbols. Power Apps text functions can do all of these things and more. In fact, according to my friend Sancho Harker they might just be the most underrated feature of Power Apps!
In this short guide I will show you how to use Power Apps text functions and give everyday examples of how you use them in your own apps.
Table of Contents:
Left, Right & Mid Functions
Find Function
Len Function
Substitute Function
Upper, Lower, Proper Functions
Concatenate Function
Char Function
TrimEnds Functions
Text Function
Other Functions
LEFT, RIGHT, MID Functions
The Left, Right and Mid functions are used to extract part of a text string.
Left( String, NumberOfCharacters )
Right( String, NumberOfCharacters )
Mid( String, StartingPosition, NumberOfCharacters )
Why I use these functions:
Companies love to use account codes to organize their records. The code below has three parts: State (NY), Year (2021) and Sequence Number (003).
"NY-2021-003"
I can get each segment of the account like this.
// get the State "NY"
Left("NY-2021-003", 2)
// get the Year "2021"
Mid("NY-2021-003", 4, 4)
// get the Sequence Number "003"
Right("NY-2021-003", 3)
FIND Function
The Find function locates the position of a text string inside another text string.
Find( FindString, WithinString [, StartingPosition ] )
Why I use this function
Suppose I have a table of employee names and want to extract the first name. I can’t use the Left or Right functions because each name has a different length.
EmployeeName |
Matthew Devaney |
Sarah Green |
Allison Reed |
By using the Find function to determine the position of the blank space in the employee name then combining it with the Left function allows me to get the first name.
Left(ThisItem.EmployeeName, Find(" ", ThisItem.EmployeeName)-1)
// Result: ["Matthew", "Sarah", "Allison"]
LEN Function
The Len function returns the length of a text string.
Len( String )
Why I use this function
Now I want to get each employee’s last name. After determining the length of the full name I can substract the position of the blank space and use the Right function to isolate only the part I want.
Right(
ThisItem.EmployeeName,
Len(ThisItem.EmployeeName)-Find(" ", ThisItem.EmployeeName)
)
// Result: ["Devaney", "Green", "Reed"]
SUBSTITUTE Function
The Substitute function replaces matching sections of a text string with another value.
Substitute( String, OldString, NewString [, InstanceNumber ] )
Why I use this function
Phone numbers are stored in a variety of different formats. In this case each block of number is separated by a dash.
PhoneNumber |
204-987-6453 |
204-998-1223 |
204-967-5834 |
If I want the phone number without the dash instead I can use the Substitute function to change the text string.
Substitute(ThisItem.PhoneNumber, "-", "")
// Result: ["2049876453", "2049981223", "2049675834"]
A more straight-forward use case for the LEN function would be to display the current length of a text string vs. its maximum size.
Len("Winnipeg1965")&"/20 characters long"
// Result: 12/20 characters long
UPPER, LOWER, PROPER Functions
The Upper, Lower and Proper functions change the case of a text string.
Lower( String )
Upper( String )
Proper( String )
Why I use these functions
When an app is opened I like to check the current user’s email to determine what role they should have (e.g. admin user, normal user). The equals operator used to check for a match is case sensitive so I convert the email to all lower case letters before making the comparison. I could have also used the Upper function to do the same thing.
User().Email
// Result: "MatthewDevaney@gmail.com"
Lower(User().Email)
// Result: "matthewdevaney@gmail.com"
Lower(User().Email)="matthewdevaney@gmail.com"
// Result: true
Another idea is to use the Proper function to capitalize the first letter of each word in a name when it is spelled in lower case.
Proper("matthew devaney")
// Result: "Matthew Devaney"
CONCATENATE Function
The Concatenate function joins together many text strings into one.
Concatenate( String1 [, String2, …] )
Why I use this function
Customer address information is typically divided into several columns when stored in a datasource (e.g. SharePoint, Dataverse).
Address | City | State | Zip Code |
10 Country Lane | Albany | NY | 90219-2003 |
67 Cherry Road | Greenville | SC | 94920-1930 |
1st Street North | Chicago | IL | 93094-3942 |
When I want to show the customer’s full address I can combine all of the columns by using the concatenate function.
Concatenate(
ThisItem.Address,
", ",
ThisItem.City,
" ",
ThisItem.State
" ",
ThisItem.'Zip Code'
)
// Result: ["10 Country Lane, Albany, NY 90219-2003", "67 Cherry Road, Greenville, SC 94920-1930", "1st Street North, Chicago, IL 93094-3942"]
CHAR Function
The Char function returns a matching ASCII character from an integer value
Char( CharacterCode )
Why I use this function
Some text strings are hard to write because they include symbols that are a a part of the Power Apps language or other tricky characters. I use the Char function to define them. Check out this page for a full list of Char codes.
// quotation marks
Char(34)&"Hello World"&Char(34)
// Result: "Hello World"
// line-break
"Hello"&Char(10)&"World"
// Result: "Hello
World"
// tabbed space
"Hello"&Char(9)&"World"
// Result: "Hello World"
TRIMENDS Function
TrimEnds removes the blank spaces from the start and end of a text string.
TrimEnds( String )
Why I use this function
Sometimes I encounter ‘dirty data’ that has extra whitepsaces and needs cleaning. The TrimEnds function makes this painless to do.
TrimEnds(" a short sentence. ")
// Result: "a short sentence"
TEXT Function
The Text function converts a number or datetime value to text and formats it.
Text( NumberOrDateTime, CustomFormat [, ResultLanguageTag ] )
Why I use this function:
A datetime value will appear in the default format found on a users device if no formatting instructions are provided. I want to control exactly what the user sees so I always define the formatting in my Power Apps. Several pre-defined formats can be found in the documentation or I can create my own custom format if needed.
// get the current time in a pre-definded format.
Text(Now(), ShortDateTime)
// Result 2/7/2021 10:34 AM
// get the current time in a custom defined format
Text(Now(), "m/d/yyyy hh:mm:ss AM/PM")
// Result 2/7/2021 10:34 AM
Another technique I use often is to format a decimal number as a currency.
// show a number with currency formatting
Text(2056.20, "$#,##0.00")
// Result $2,056.00
Other Functions
There are a few more text functions I use less often. If you want to check them out you click on one links below and it will take you to the Power Apps formulas reference.
Did You Enjoy This Article? 😺
Subscribe to get new Power Apps articles sent to your inbox each week for FREE
Questions?
If you have any questions or feedback about Power Apps Text Functions (With Examples) please leave a message in the comments section below. You can post using your email address and are not required to create an account to join the discussion.
Great stuff, again. I had forgotten about Proper(). I like how you have them all laid out with real-life examples. Perhaps a new blog post for more complex uses? For example, using substitute to recursively replace non-alphanumeric characters in a variable? (I actually know how to do it, but it took a lot of work to figure out.
Valerie,
Sounds like a fun challenge. I’ve already started puzzling through it in my head. Why not write a blog of your own if you have already figured it out and share your knowledge with the world?
That would require a blog to post in…
Hi Matt! Here’s the code for recursively replacing various symbols. There may be a better way, but it’s the one I got to work. This requires the variable varText to already contain a string.
I got a very good refreshing. Thanks, Matthew
Hello Matthew,
I tried your Char example for the quotation marks but ended up with ‘/’ symbol. After a quick research, I found that the correct Char code for quotation is Char(47).
.. and thanks for you great posts.
Ahmed
Ahmed,
You are definitely correct that the quotation marks Char code Char(47) is wrong. The correct Char code for double-quotation marks is Char(34). I have updataed my article. Thank you.
thanks alot of information
Hi Matt, I’m trying to use the “Left” and “Find” function like what you showed above where you used “Left(ThisItem.EmployeeName, Find(” “, ThisItem.EmployeeName)-1)”. Every time I add the “-1” to the find portion of the formula, Power Apps complains saying “The second argument of the ‘Left’ function is invalid yet the formula works. The issue is when I run the app, it pops up the same error message. Note I’m putting this formula within a gallery item control. Here is my exact formula:
If(Left(ThisItem.’Link to item’,Find(“?”,ThisItem.’Link to item’)-1)=varCurrentProject.’Link to FORM’,” (*Main Form)”,””)
Any ideas?
What are the parameters of the Replace function, and how do they affect its functionality?
Depludi,
Here is a link to the document for REPLACE: https://learn.microsoft.com/en-us/power-platform/power-fx/reference/function-replace-substitute
Is there a workaround to the Left, Mid, and Right functions if I keep getting a delegation warning? Specifically, I am trying to extract the last 8 digits of a string, so I am using Right(field99, 8) within a lookup/filter. I am trying to create a local collection with this lookup from my SharePoint data source.
Gabriella,
Yes, there is a PowerFx function workaround for Left. It is the StartsWith function.
No, there is are no PowerFx function workarounds for Right and Mid. You would need to have a new column in your database holding only the Right, Mid characters and do an equals comparison.
Hello Matthew,
for Power Apps text input field, how to create a line break for each line in the paragraph? the requirement is to assign a number of lines for each text input, then the user should have to stop at the maximum line. each line should have to count. My code counts a paragraph as one line. Thank you.