25 Power Apps IF Function Examples
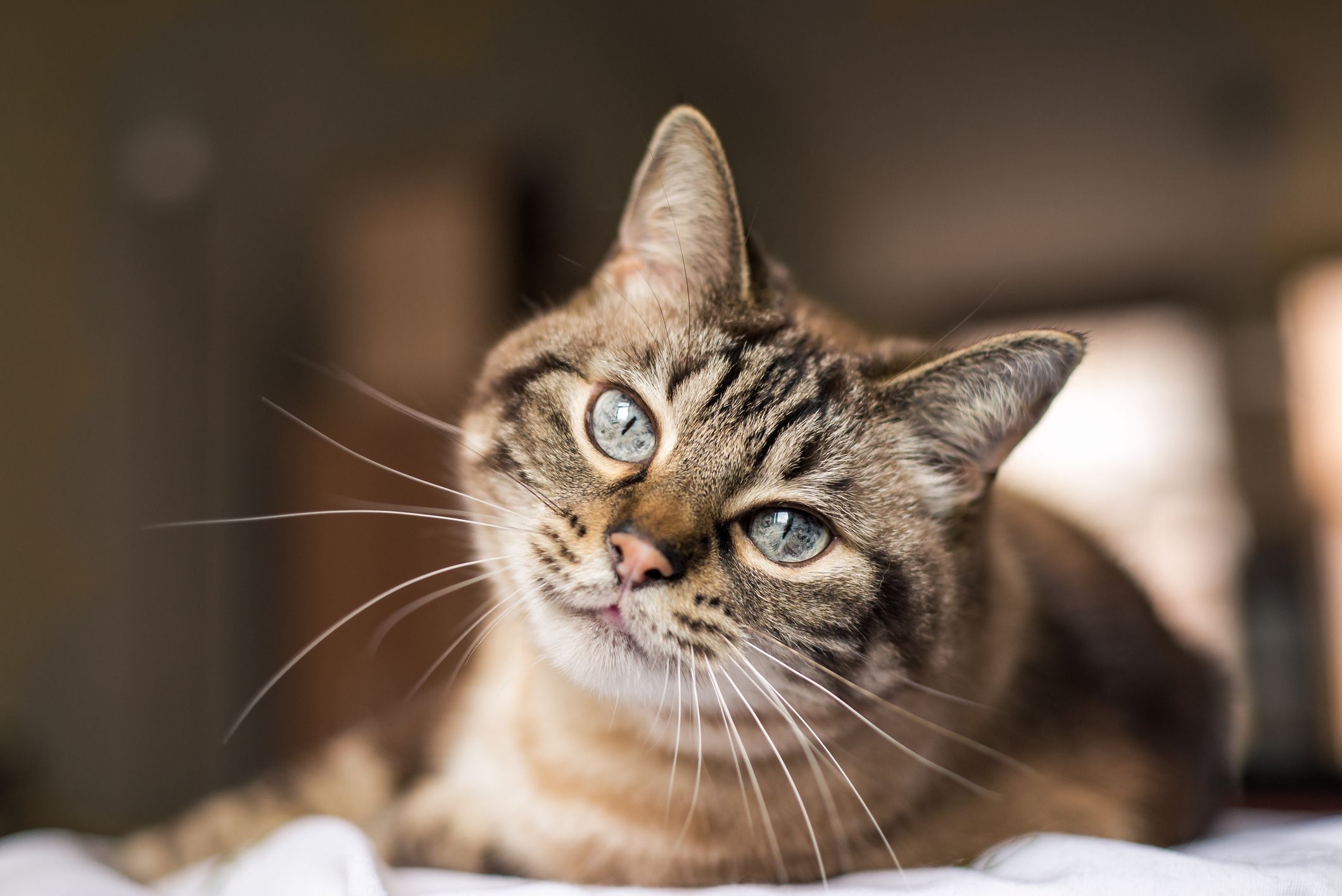
The Power Apps IF Function performs a logical comparison to see if the result is true. Then it conditionally executes the next lines of code based on whether the result was true or false. The examples on this page show how the Power Apps IF Function works for every data type.
Important note: for most examples the IF Function the 2nd argument is true and the 3rd argument is false. These true and false values are only placeholders. They should be replaced with your own code in the real-world.
Table of Contents
• IF Function Text Examples In Power Apps
◦ IF Text Value Equals
◦ IF Text Value Contains
◦ IF Text Value StartsWith
◦ IF Text Value Is Blank
◦ IF Text Value Equals With Multiple Conditions
• IF Function Number Examples In Power Apps
◦ IF Number Value Is Greater Than Or Less Than
◦ IF Number Value Is Greater Than Or Equal To/Less Than Or Equal To
◦ IF Number Value Does Not Equal
◦ IF Number Value Is Between Two Values
◦ IF Number Is Found Within A Range (Multiple Conditions)
• IF Function Date Examples In Power Apps
◦ IF Date Value Equals Current Date
◦ IF Date Value Equals A Specific Date
◦ IF Date Value Is Between Two Dates
• IF Function Boolean Examples In Power Apps
◦ IF Boolean Value Equals true
◦ IF Boolean Value Equals false
• IF Function Choice Column Examples In Power Apps
◦ IF Choice Column Value Equals
• IF Function LookUp Column Examples In Power Apps
◦ IF LookUp Column ID Equals
◦ IF LookUp Column Value Equals
• IF Function Person Column Examples In Power Apps
◦ IF Person Column Equals Current User
◦ IF Person Column Equals User Name
◦ IF Person Column Equals User Email
• Additional IF Function Examples For Power Apps
◦ IF Condition Using AND Logical Operator
◦ IF Condition Using OR Logical Operator
◦ IF Condition Using NOT Logical Operator
◦ IF Condition Using Multiple Logical Operators
IF Function Text Examples In Power Apps
IF Text Value Equals
Input:
Set(varManufacturer, "Samsung")
Code:
Check if varManufacturer equals Samsung
If(varManufacturer ="Samsung", true, false)
Output:
true
IF Text Value Contains
Input:
Set(varTitle, "Dishwasher")
Code:
Check if varTitle contains “wash”
If("wash" in varTitle, true, false)
Output:
true
IF Text Value StartsWith
Input:
Set(varTitle, "Kitchen Sink")
Code:
Check if varTitle starts with “Kitchen”
If(StartsWith(Title, "Kitchen"), true, false)
Output:
true
IF Text Value Is Blank
Input:
Set(varManufacturer, Blank())
Code:
Check if varManufacturer is blank
If(IsBlank(varManufacturer), true, false)
Output:
true
IF Text Value Equals With Multiple Conditions
Input:
Set(varTitle, "Kitchen Sink")
Code:
Check multiple conditions and return the result where varTitle is equal to “Kitchen Sink”
If(
varTitle = "Dishwasher",
"Dishwasher was found!",
varTitle = "Kitchen Sink",
"Kitchen Sink was found!",
varTitle = "Refrigerator",
"Refrigerator was found!",
"No matches found"
)
Output:
"Kitchen Sink was found!"
IF Function Number Examples In Power Apps
IF Number Value Is Greater Than Or Less Than
Input:
Set(varQuantityInStock, 15)
Code:
Check if varQuantityInStock is greater than 10
If(varQuantityInStock > 10, true, false)
Output:
true
IF Number Value Is Greater Than Or Equal To/Less Than Or Equal To
Input:
Set(varQuantityInStock, 8)
Code:
Check if varQuantityInStock is less than or equal to 10
If(varQuantityInStock <= 10, true, false)
Output:
true
IF Number Value Does Not Equal
Input:
Set(varQuantityInStock, 2)
Code:
Check if varQuantityInStock does not equal 9
If(varQuantityInStock <> 9, true, false)
Output:
true
IF Number Value Is Between Two Values
Input:
Set(varQuantityInStock, 6)
Code:
Check if varQuantityInStock is between 5 and 10
If(
varQuantityInStock >= 5
And varQuantityInStock <= 10,
true,
false
)
Output:
true
IF Number Is Found Within A Range (Multiple Conditions)
Input:
Set(varQuantityInStock, 5)
Code:
Check multiple conditions and return the result where varQuantityInStock is greater than 0 but less than 10.
If(
varQuantityInStock >= 50,
"Too many in stock",
varQuantityInStock >= 10,
"Item is in stock",
varQuantityInStock > 0,
"Low inventory, time to re-stock",
"No inventory left"
)
Output:
"Low inventory, time to re-stock"
IF Function Date Examples In Power Apps
IF Date Value Equals Current Date
Input:
Set(varLastSoldDate, Today())
Code:
Assuming the current date is March 6, 2023, check if varLastSoldDate equals today
If(varLastSoldDate = Today(), true, false)
Output:
true
IF Date Value Equals A Specific Date
Input:
Set(varLastSoldDate, Date(2023, 3, 1))
Code:
Check if LastSoldDate column equals March 1, 2023
If(varLastSoldDate = Date(2023, 3, 1), true, false)
Output:
true
IF Date Value Is Between Two Dates
Input:
Set(varLastSoldDate, Date(2023, 3, 10))
Code:
Check if varLastSoldDate is between March 1, 2023 and March 31, 2023
If(
varLastSoldDate >= Date(2023, 3, 1)
And varLastSoldDate <= Date(2023, 3, 31),
true,
false
)
Output:
true
IF Function Boolean Examples In Power Apps
Boolean Value Equals true
Input:
Set(varOnSale, true)
Code:
Check if varOnSale equals true
If(varOnSale, true, false)
Output:
true
IF Boolean Value Equals false
Input:
Set(varOnSale, false)
Code:
Check if varOnSale equals false
If(!varOnSale, true, false)
Output:
true
IF Function Choice Column Examples In Power Apps
IF Choice Column Value Equals
Input:
Inventory SharePoint List
ID | Title | OrderStatus |
1 | Dishwasher | Ordered |
2 | Freezer | Ordered |
3 | Kitchen Faucet | Not Ordered |
4 | Kitchen Sink | Discontinued |
5 | Refrigerator | Ordered |
6 | Stove | Not Ordered |
Get the first row in the Inventory SharePoint list where OrderStatus is a Choice type column
Set(varInventoryItem, LookUp(Inventory, ID=1))
Code:
Check if the OrderStatus of varInventoryItem equals “Ordered”
If(varInventoryItem.OrderStatus.Value = "Ordered", true, false)
Output:
true
IF Function LookUp Column Examples In Power Apps
IF LookUp Column ID Equals
Input:
Inventory SharePoint List
ID | Title | Manufacturer |
1 | Dishwasher | LG |
2 | Freezer | Samsung |
3 | Kitchen Faucet | Kohler |
4 | Kitchen Sink | American Standard |
5 | Refrigerator | Samsung |
6 | Stove |
Manufacturers SharePoint List
ID | Title |
1 | LG |
2 | Samsung |
3 | Kohler |
4 | American Standard |
Get the 3rd row in the Inventory SharePoint list where Manufacturer is a Lookup type column
Set(varInventoryItem, LookUp(Inventory, ID=3))
Code:
Check if the ID of varInventoryItem equals 3
If(Manufacturer.ID = 3, true, false)
Output:
true
IF LookUp Column Value Equals
Input:
Inventory SharePoint List
ID | Title | Manufacturer |
1 | Dishwasher | LG |
2 | Freezer | Samsung |
3 | Kitchen Faucet | Kohler |
4 | Kitchen Sink | American Standard |
5 | Refrigerator | Samsung |
6 | Stove |
Manufacturers SharePoint List
ID | Title |
1 | LG |
2 | Samsung |
3 | Kohler |
4 | American Standard |
Get the 4th row in the Inventory SharePoint list where Manufacturer is a Lookup type column
Set(varInventoryItem, LookUp(Inventory, ID=4))
Code:
Check if the Title of varInventoryItem equals “American Standard”
If(Manufacturer.Value = "American Standard", true, false)
Output:
true
IF Function Person Column Examples In Power Apps
IF Person Column Equals Current User
Input:
Inventory SharePoint List
ID | Title | Buyer |
1 | Dishwasher | Matthew Devaney |
2 | Freezer | Sarah Green |
3 | Kitchen Faucet | Matthew Devaney |
4 | Kitchen Sink | David Johnson |
5 | Refrigerator | David Johnson |
6 | Stove | Alice Lemon |
Get the first in the Inventory SharePoint list where Buyer is a Person type column
Set(varInventoryItem, LookUp(Inventory, ID=1))
Code:
Assuming the current user is Matthew Devaney, check if the Buyer of varInventoryItem equals the current user
If(varInventoryItem.Buyer.Email = User().Email, true, false)
Output:
true
IF Person Column Equals User Name
Input:
Inventory SharePoint List
ID | Title | Buyer |
1 | Dishwasher | Matthew Devaney |
2 | Freezer | Sarah Green |
3 | Kitchen Faucet | Matthew Devaney |
4 | Kitchen Sink | David Johnson |
5 | Refrigerator | David Johnson |
6 | Stove | Alice Lemon |
Get the 2nd row in the Inventory SharePoint list where Buyer is a Lookup type column
Set(varInventoryItem, LookUp(Inventory, ID=2))
Code:
Check if the Buyer column of varInventoryItem has a user name equal to “Sarah Green”
If(varInventoryItem.Buyer.'Display Name = "Sarah Green", true, false)
Output:
true
IF Person Column Equals User Email
Input:
Inventory SharePoint List
ID | Title | Buyer |
1 | Dishwasher | Matthew Devaney |
2 | Freezer | Sarah Green |
3 | Kitchen Faucet | Matthew Devaney |
4 | Kitchen Sink | David Johnson |
5 | Refrigerator | David Johnson |
6 | Stove | Alice Lemon |
Get the 5th row in the Inventory SharePoint list where Buyer is a Person type column
Set(varInventoryItem, LookUp(Inventory, ID=5))
Code:
Check if the Buyer column of varInventoryItem has an email equal to “[email protected]”
If(varInventoryItem.Buyer.Email = "[email protected]")
Output:
true
Additional IF Function Examples For Power Apps
IF Condition Using AND Logical Operator
Input:
Inventory SharePoint List
ID | Title | Manufacturer | OnSale |
1 | Dishwasher | LG | Yes |
2 | Freezer | Samsung | No |
3 | Kitchen Faucet | Kohler | No |
4 | Kitchen Sink | American Standard | No |
5 | Refrigerator | Samsung | Yes |
6 | Stove | No |
Get the 1st row in the Inventory SharePoint list where Manufacturer is a text column and OnSale is a Yes/No type column
Set(varInventoryItem, LookUp(Inventory, ID=5))
Code:
Check if varInventoryItem has a Manufacturer equal to “Samsung” and an OnSale column equal to Yes.
If(
varInventoryItem.Manufacturer="Samsung"
And varInventoryItem.OnSale,
true,
false
)
Output:
true
IF Condition Using OR Logical Operator
Input:
Inventory SharePoint List
ID | Title | Manufacturer | OnSale |
1 | Dishwasher | LG | Yes |
2 | Freezer | Samsung | No |
3 | Kitchen Faucet | Kohler | No |
4 | Kitchen Sink | American Standard | No |
5 | Refrigerator | Samsung | Yes |
6 | Stove | No |
Get the 2nd row in the Inventory SharePoint list where Manufacturer is a text column and OnSale is a Yes/No type column.
Set(varInventoryItem, LookUp(Inventory, ID=2))
Code:
Check if varInventoryItem has a Manufacturer equal to “Samsung” or an OnSale column equal to Yes.
If(
varInventoryItem.Manufacturer="Samsung"
Or varInventoryItem.OnSale,
true,
false
)
Output:
true
IF Condition Using NOT Logical Operator
Input:
Inventory SharePoint List
ID | Title | Manufacturer | OnSale |
1 | Dishwasher | LG | Yes |
2 | Freezer | Samsung | No |
3 | Kitchen Faucet | Kohler | No |
4 | Kitchen Sink | American Standard | No |
5 | Refrigerator | Samsung | Yes |
6 | Stove | No |
Get the 3rd row in the Inventory SharePoint list where Manufacturer is a text column and OnSale is a Yes/No type column.
Set(varInventoryItem, LookUp(Inventory, ID=3))
Code:
Check if varInventoryItem has a Manufacturer column not equal to “Samsung”
If(Not(varInventoryItem="Samsung"), true, false)
Output:
true
IF Condition Using Multiple Logical Operators
Input:
Inventory SharePoint List
ID | Title | Manufacturer | OnSale |
1 | Dishwasher | LG | Yes |
2 | Freezer | Samsung | No |
3 | Kitchen Faucet | Kohler | No |
4 | Kitchen Sink | American Standard | No |
5 | Refrigerator | Samsung | Yes |
6 | Stove | No |
Get the 1st row in the Inventory SharePoint list where Manufacturer is a text column and OnSale is a Yes/No type column
Set(varInventoryItem, LookUp(Inventory, ID=1))
Code:
Check if varInventoryItem has a Manufacturer column equals “Samsung” and OnSale equals Yes or the Manufacturer column equals “LG”
If(
(
varInventoryItem.Manufacturer="LG"
And varInventoryItem.OnSale="Yes"
)
Or varInventoryItem.Manufacturer="LG"),
true,
false
)
Output:
true
Did You Enjoy This Article? 😺
Subscribe to get new Power Apps articles sent to your inbox each week for FREE
Questions?
If you have any questions or feedback about 25 Power Apps IF Function Examples please leave a message in the comments section below. You can post using your email address and are not required to create an account to join the discussion.
I try to explain to all new powerapps developers or citizens that writing “if(my condition,true,false)” is useless and make the readability of code worse.
Can you tell us if there is a real need of this syntax when writing just “myCondition” in a Boolean field is enough ?
Alexsolex,
Agreed that when your IF function evaluates to either true or false you should skip it and just write varTextValue=”ABC” instead of If(varTextValue=”ABC”, true, false).
What I am trying to focus on with my examples is how to write conditions within the IF Function’s first argument. My thinking was if the reader understands how to write conditions, then they could fill-in the true and false outcomes with their own value. Let’s see what feedback is received on this article. If I have assumed too much knowledge of the reader then I will revise it.
As always, I appreciate the feeback Alexsolex 🙂
Hi Matthew. Really handy list, thank you.
I’m not sure if you also do this, but when I have a conditional with OR like this:
If(varInventoryItem=”Samsung” Or varInventoryItem=”LG” , true, false)
I prefer to write it as:
If(varInventoryItem in [“Samsung” , “LG”] , true, false)
as it makes it easier to read and to add more items to the list (in this case, of Manufacturers).
What do you think?
Cheers,
Andrea
Andrea,
Yes, I do use this method… a lot! Thank you for reminding me about it. I will update my article later this week to include. You rock 🎸
Hi Matthew, I tried to modify nested if in power automate following what is explained on this article and could not save the compose action….
First, i created Initialize variable action of string type ‘varTransport’ and the value is based on a Msforms Input
Then a compose action : if(“Train” in variables(‘varTransport’),true,”Autobus” in variables(‘varTransport’),true,”Location automobile” in variables(‘varTransport’),true,false)
Am I missing something ?
Thanks,
Jerome,
This article shows code examples for Power Apps. You appear to be asking about Power Automate. Is it possible you have misread my article and believed it was for Power Automate?
I wish this article had of existed when I started my PowerApps journey 2 years ago. It would have saved me many tears. Especially the logical operators section. Will be sharing this with the up and comers I am mentoring.
Heidi,
I am glad to hear I am writing the content you wished existed. But the better news to me is, you will share it with the dev you are mentoring
Having a good mentor is important. Thanks for doing what you do and helping others grow.
Hi Matthew:
Thanks for this – did not realize that there was a switch style statement as you showed in Check multiple conditions!
cheers
Drew,
Just a quick note on this. It only executes code for the first logical comparison that passes the true/false check. If multiple logical comparisons pass it still only executes the first. Thanks for dropping me a comment 🙂
Matthew,
I’m a huge fan of your work. Thanks for everything you do.
In reading the article, I had a question:
Code:
Check if the Buyer column of varInventoryItem has a user name equal to “Sarah Green”
If(varInventoryItem = Buyer.’Display Name’, true, false)
Wouldn’t it be as follows?:
If(varInventoryItem.Buyer.’Display Name’ = “Sarah Green”, true, false)
Best,
Pete
Pete,
Good catch! I agree that requires an update.
Check if the OrderStatus of varInventoryItem equals “Ordered”
should this code like below
thank you for your great posting
LDK,
Good catch. Thank you 😊
I think this Output is not ok:
Fernando,
Excellent find. It’s now fixed.
I tried this syntax but did not get the desired result. I ended up using a switch.
Also, it works if I treat it with nested If statements. Has something changed since you wrote this?
If(
varTitle = “Dishwasher”,
“Dishwasher was found!”,
varTitle = “Kitchen Sink”,
“Kitchen Sink was found!”,
varTitle = “Refrigerator”
“Refrigerator was found!”,
“No matches found”
)
Oscar,
It’s missing a comma after “Refrigerator.” I’ll go fix that now 😉
Hi @Matthew,
Sorry for late reply, correct me if I am wrong but this code that you on example shouldn’t return false?
Regards
Lütfi,
You’re correct. I’ve fixed it now. Thanks!
If(
IsBlank(DataCardValue35.SelectedItems),Set(varTask,true),
IsBlank(DataCardValue1.SelectedItems),Set(varApplicationArea,true),
IsBlank(DataCardValue36.SelectedItems),Set(varTimein,true),
IsBlank(DataCardValue40.SelectedItems),Set(varTimeout,true),
TimeValue( DataCardValue36.Selected.Value)>=TimeValue(DataCardValue40.Selected.Value), Set(varLabel11, true),Set(varLabel11, false) Or
IsBlank(DataCardValue8.SelectedItems),Set(varTask,true),
IsBlank(DataCardValue2.SelectedItems),Set(varApplicationArea,true),
IsBlank(DataCardValue4.SelectedItems),Set(varTimein,true),
IsBlank(DataCardValue5.SelectedItems),Set(varTimeout,true),
TimeValue( DataCardValue4.Selected.Value)>=TimeValue(DataCardValue5.Selected.Value), Set(varLabel11, true),Set(varLabel11, false),
IsBlank(DataCardValue10.SelectedItems),Set(varTask,true),
IsBlank(DataCardValue3.SelectedItems),Set(varApplicationArea,true),
IsBlank(DataCardValue14.SelectedItems),Set(varTimein,true),
IsBlank(DataCardValue15.SelectedItems),Set(varTimeout,true),
TimeValue( DataCardValue14.Selected.Value)>=TimeValue(DataCardValue15.Selected.Value), Set(varLabel11, true),Set(varLabel11, false),
IsBlank(DataCardValue7.SelectedItems),Set(varTask,true),
IsBlank(DataCardValue17.SelectedItems),Set(varApplicationArea,true),
IsBlank(DataCardValue29.SelectedItems),Set(varTimein,true),
IsBlank(DataCardValue24.SelectedItems),Set(varTimeout,true),
TimeValue( DataCardValue29.Selected.Value)>=TimeValue(DataCardValue24.Selected.Value), Set(varLabel11, true),Set(varLabel11, false),
This is my if statement but its not working says can’t evaluate formula. I have 4 forms and in each I want to validate if the forms aint blank and that time in and time out can’t be the same. Please help