Power Apps OnError Property: Capture & Log Unexpected Errors
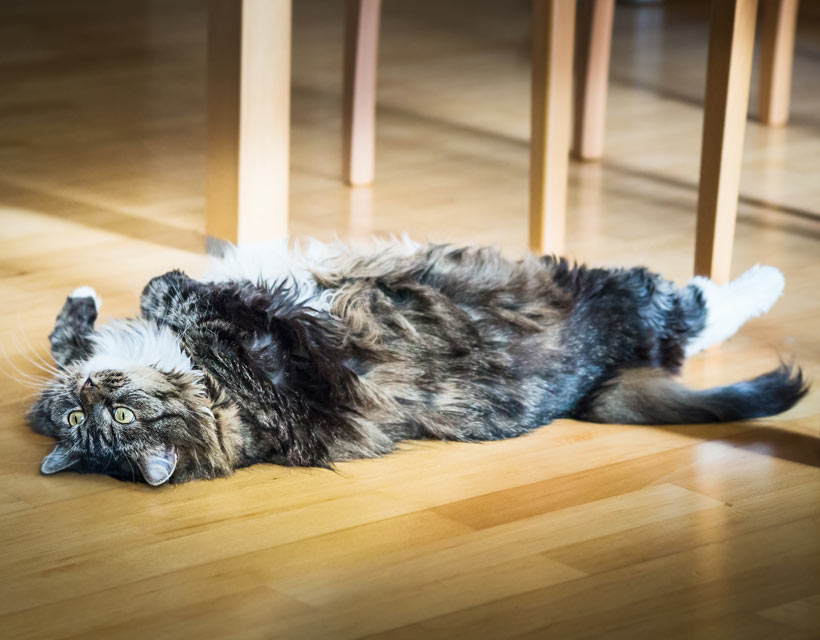
As an app developer I try to anticipate any possible errors the user could get and tell the app what to do when that error happens. But I can’t anticipate every possible scenario, so what should I do in those cases? With Power Apps OnError property we can tell the app to display a custom message for any unhandled errors, send details to the app monitor and record the errors in a log file for the developers to take a look at later.
In this tutorial I will explain the new Power Apps OnError Property and show your best way to deal with unhandled errors.
Table Of Contents
• Introduction: The Currency Exchange Calculator App
• Enable The Formula-Level Error Management Setting
• Create A New Canvas App In Power Apps Studio
• Calculate The Currency Exchange Rate
• Generate An Unhandled Error In Power Apps
• Write A Custom Error Message With Power Apps OnError Property
• Send Error Details To Power Apps Monitor
• Track Unhandled Power Apps Errors In Application Insights
• Link The Power Apps App To Application Insights
• Get A Report Of All Power Apps Errors In Application Insights
• List Of Power Apps Error Kind Codes
Introduction: The Currency Exchange Calculator App
The Currency Exchange Calculator App is used by employees at a travel agency to check the current rates for clients. When an employee inputs an invalid value into the app they see an error message on the screen and the error details are silently logged to a database for the application development team to review.
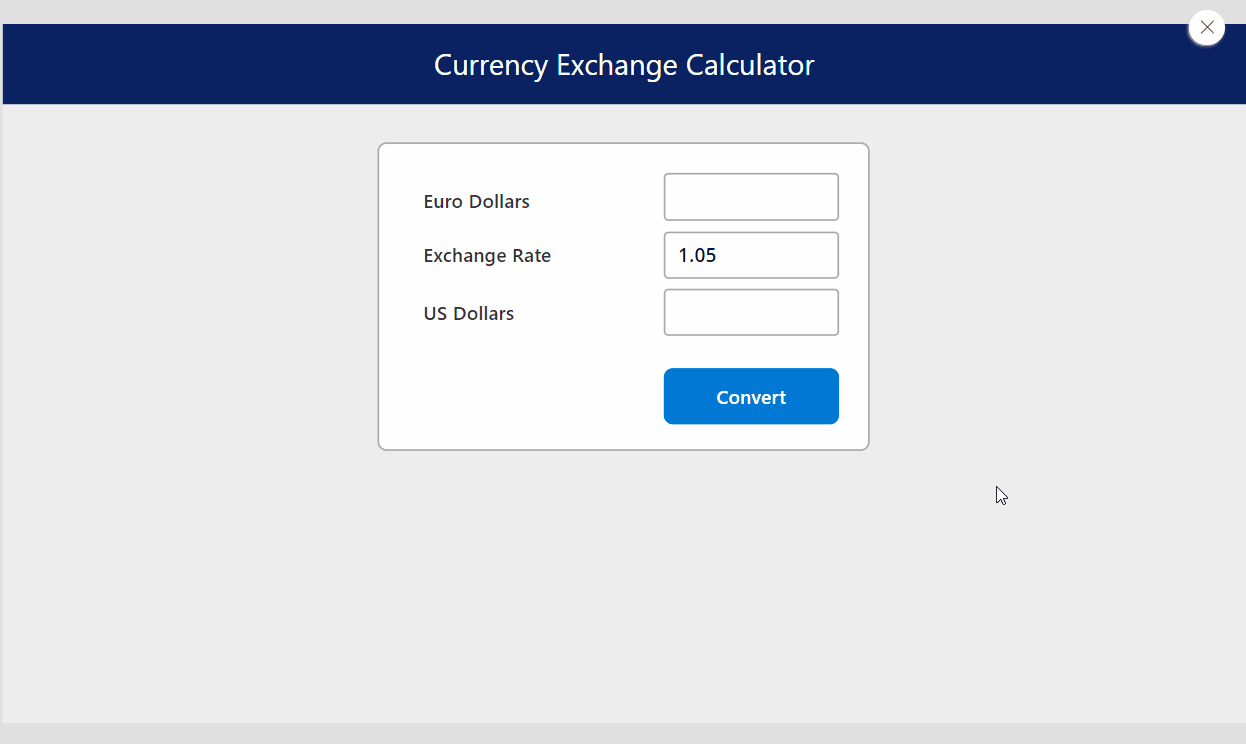
Enable The Formula-Level Error Management Setting
The new Power Apps OnError property is still in experimental mode. To use this new feature open Power Apps Studio and create a new canvas app from blank. Then go to File > Settings > Upcoming features > Experimental and turn on Formula-level error management.
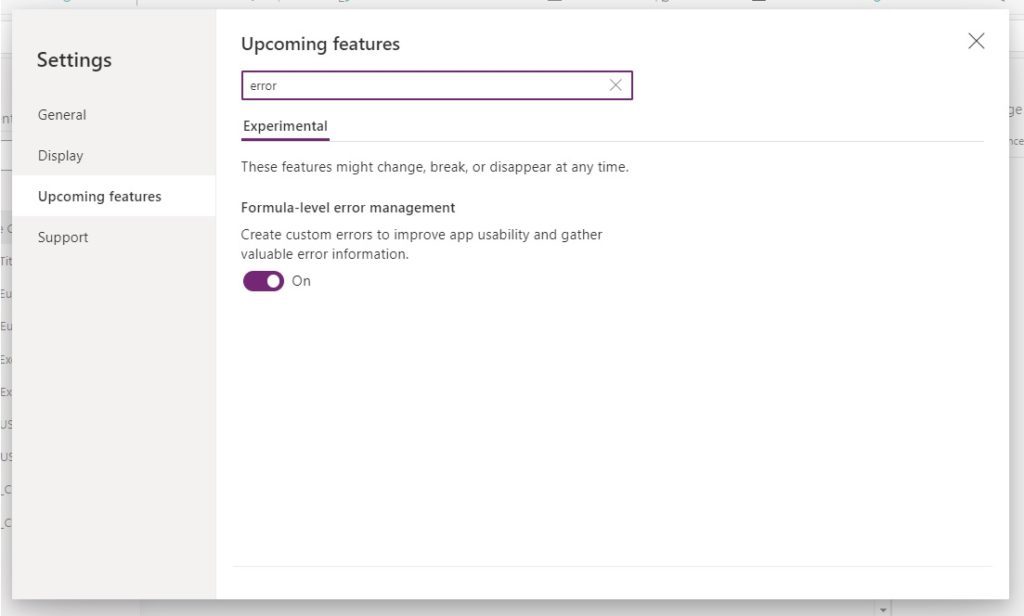
Also make sure that the Power Apps Authoring version is 3.21122 or greater.
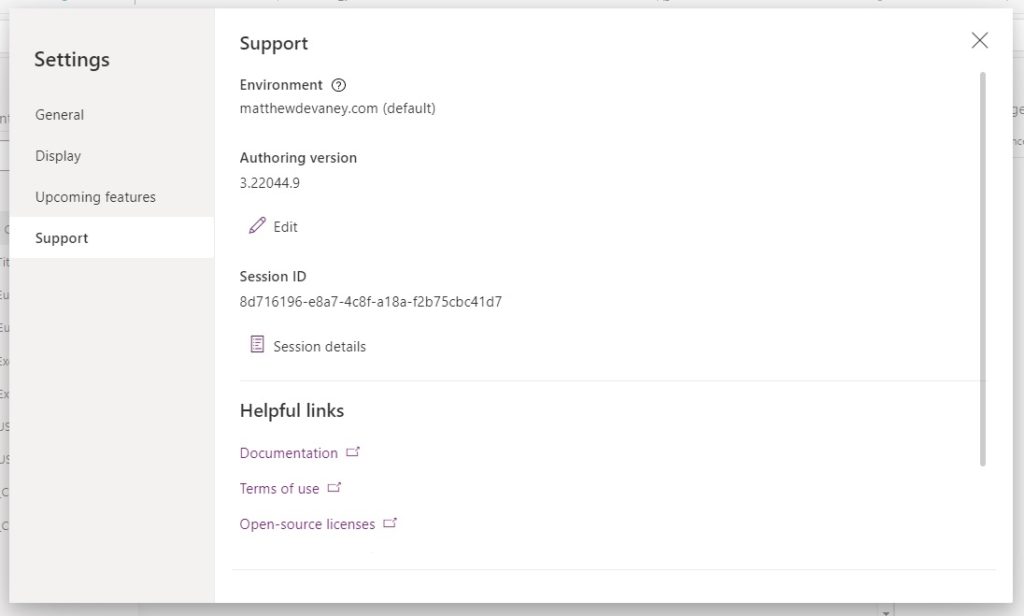
Create A New Canvas App In Power Apps Studio
In our newly created canvas app, insert a label with the text “Currency Exchange Calculator” at the top of the screen.
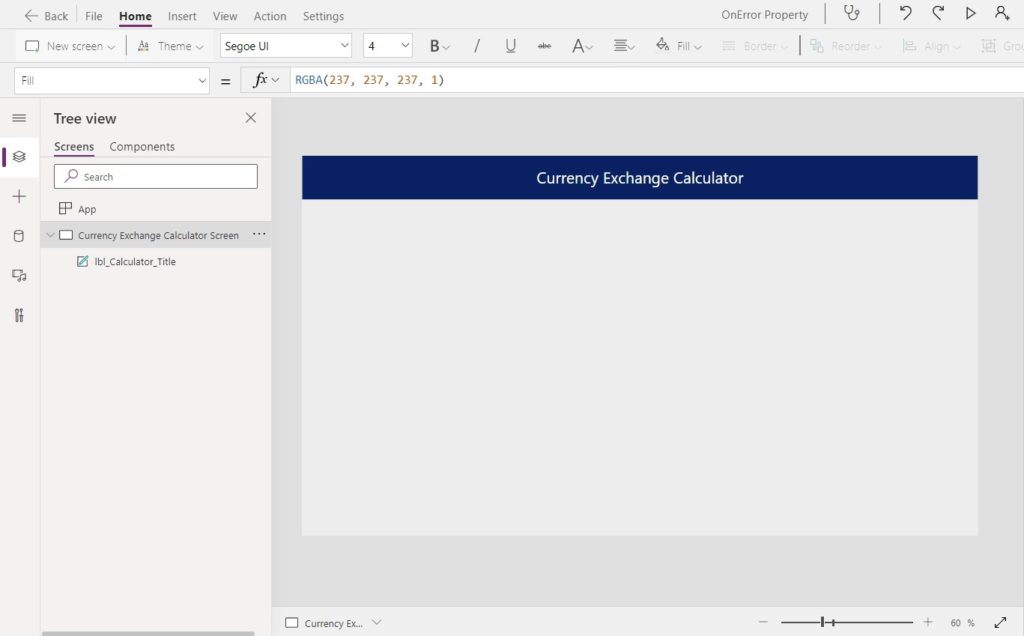
Next, place a button onto the screen. We will change its style to look like a card and then position several other controls on top of it.
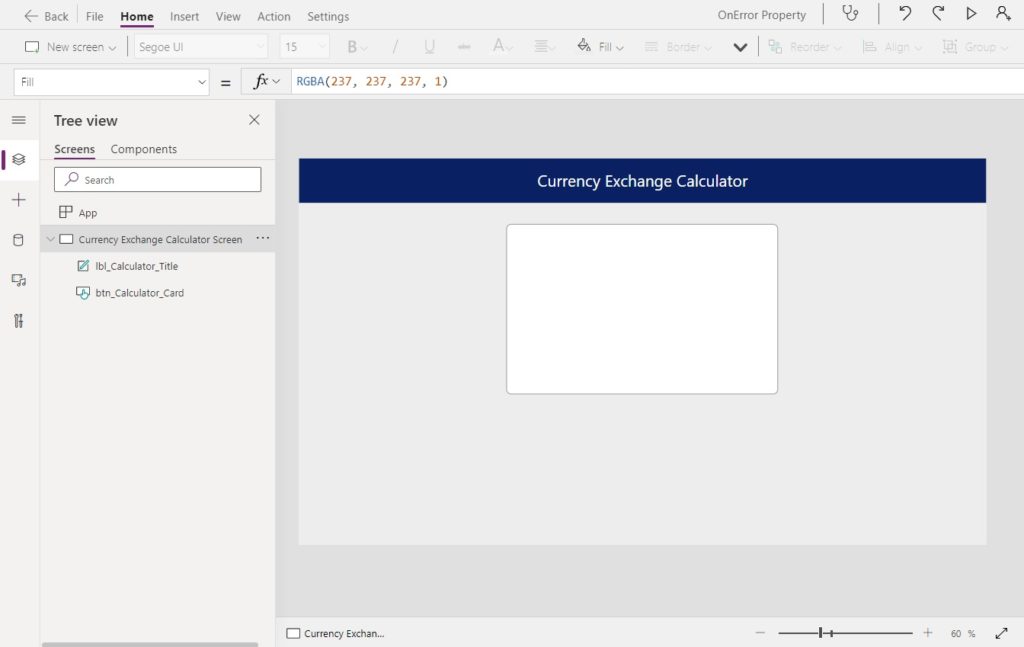
Use these settings in the following properties of the button.
BorderRadiusBottomLeft: 10
BorderRadiusBottomRight: 10
BorderRadiusTopLeft: 10
BorderRadiusTopRight: 10
DisabledBorderColor: DarkGray
DisplayMode: DisplayModel.Disabled
Fill: White
Then create three pairings of labels and text inputs as shown in the image below and place a button at the bottom:
- Euro Dollars
- Exchange Rate
- US Dollars
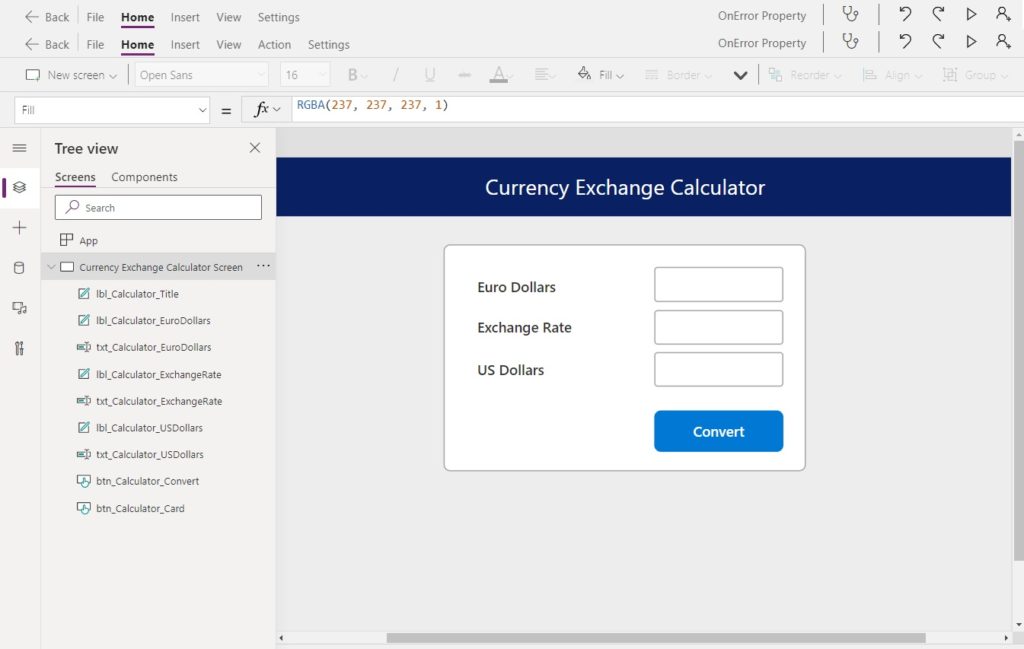
Calculate The Currency Exchange Rate
With basic design of our app completed, next we will add some code to calculate the currency exchange rate. Our goal is to generate an error message using Power Apps OnError Property so we will intentionally make a mistake. Select the Euro Dollars text input and change the Format property to Text. This is wrong because only numbers should be written in the text input.
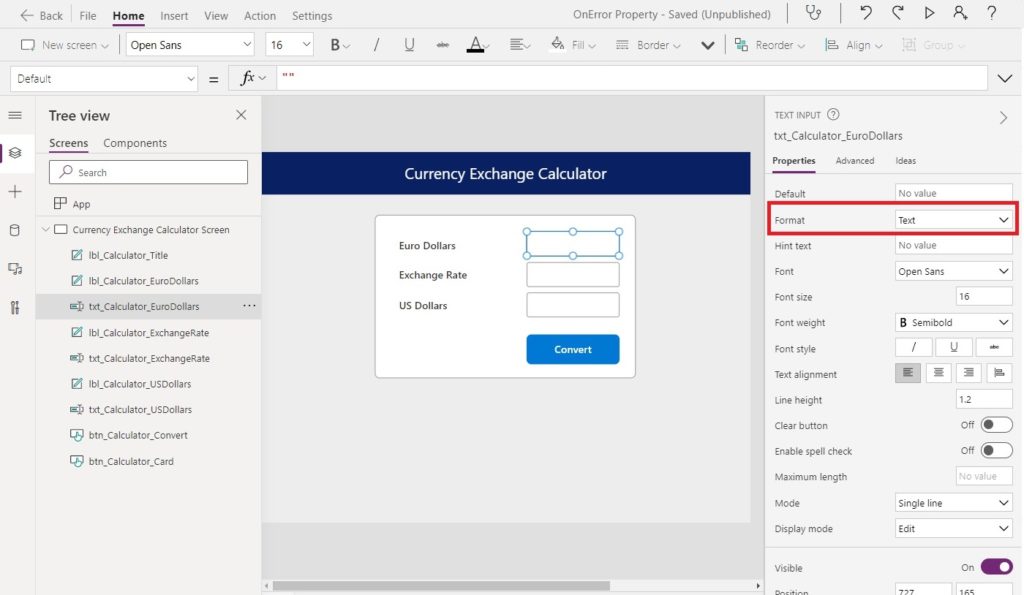
Then write the value 1.05 in the Default property of the Exchange Rate text input. This is a hardcoded value but if we were making a real app the exchange rate would be filled-in from an online service or some other datasource.
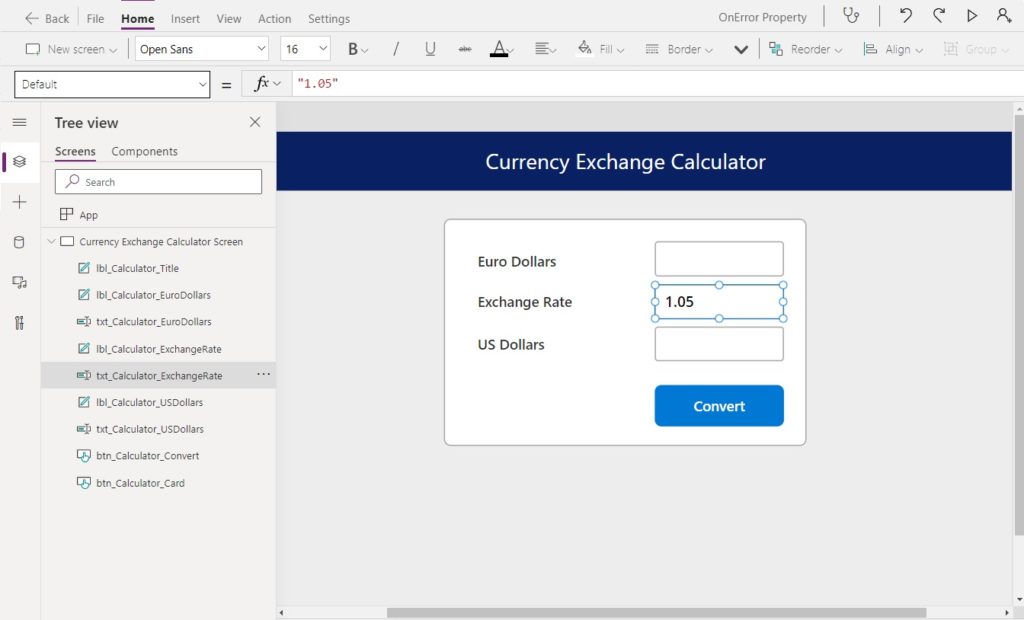
When the Convert button is clicked it will multiply the amount of Euro Dollars by the exchange rate and store the result in a variable.
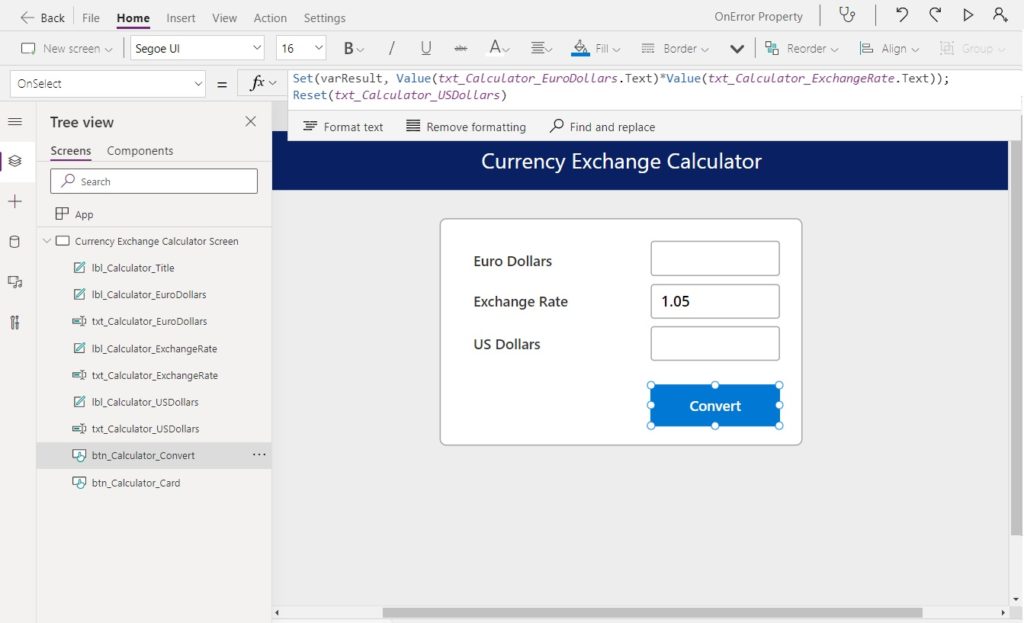
Use this code in the OnSelect property of the Convert button.
Set(
varResult,
Value(txt_Calculator_EuroDollars.Text)
* Value(txt_Calculator_ExchangeRate.Text)
);
Reset(txt_Calculator_USDollars)
The result of the currency exchange calculation should be displayed in the US Dollars text input.
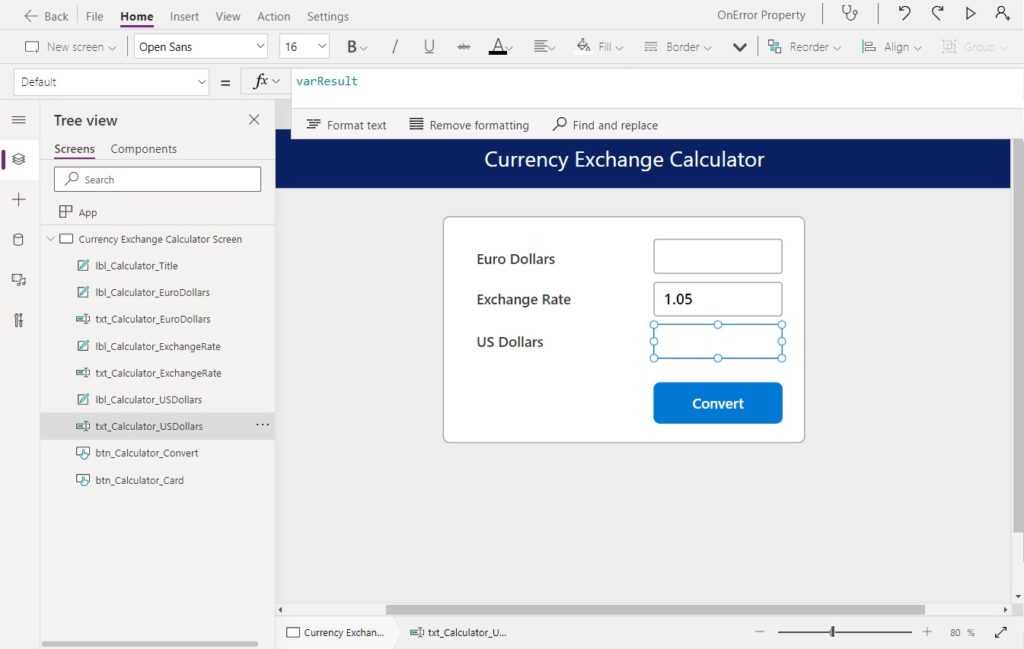
Use this code in the Default property of the US Dollars text input.
varResult
Generate An Unhandled Error In Power Apps
Our goal was to create an app that would generate an error message. Now we have the ability to do that. Write the letters “ABC” in the Euro Dollars text input and click the Convert button. When we do this Power Apps will display an error message that says “Invalid arguments to the Value function”. The Value function converts a text string to a number. An error occurs because ABC cannot be converted to a number.
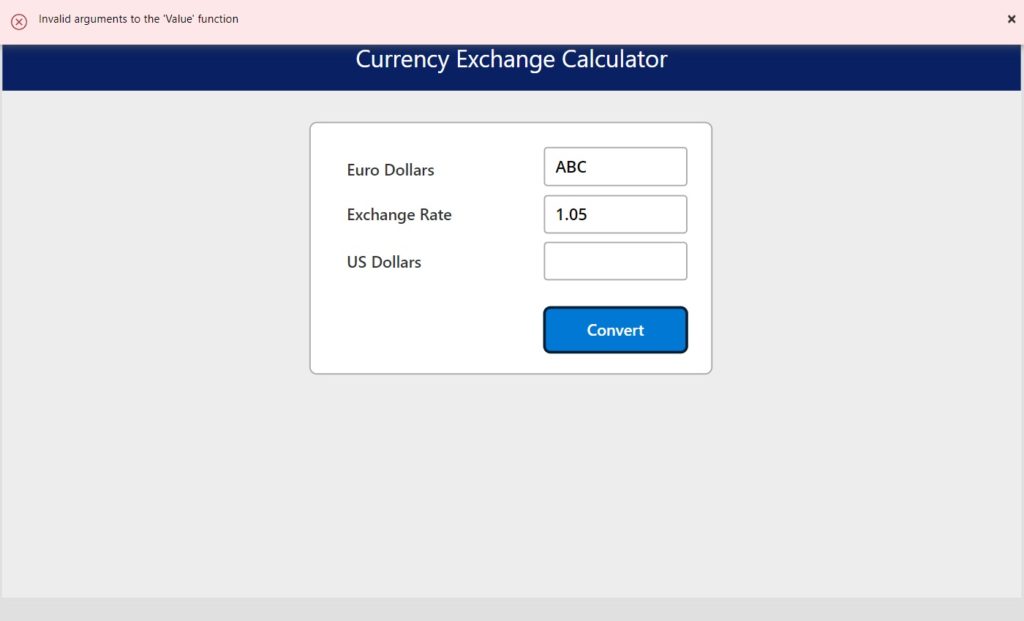
The error that we generated is considered an unhandled error. When an unhandled error occurs Power Apps checks the OnError property for what actions to take next, usually, display a custom error message and trace the error details. Right now we do not have and logic written in Power Apps OnError property so it displays a generic error message by default.
A handled error is one that the developer anticipated in advance and wrote specific logic in an IfError function to tell Power Apps what should happen next. For example, we could have written our button’s On Select button code like this. The 1st argument to IfError is a set of code Power Apps should try to execute. If that code fails for any reason Power Apps will run the code in IfError’s 2nd argument.
IMPORTANT: Do not change the button’s OnSelect code to use IfError. The rest of this tutorial will not work if you do this. |
IfError(
Set(
varResult,
Value(txt_Calculator_EuroDollars.Text)
* Value(txt_Calculator_ExchangeRate.Text)
);
Reset(txt_Calculator_USDollars),
Notify(
"An error occurred: " & FirstError.Message,
NotificationType.Error
)
);
Write A Custom Error Message With Power Apps OnError Property
When an unhandled error happens we should use Power Apps OnError property to give a detailed error message that will allow the developer to debug the app.
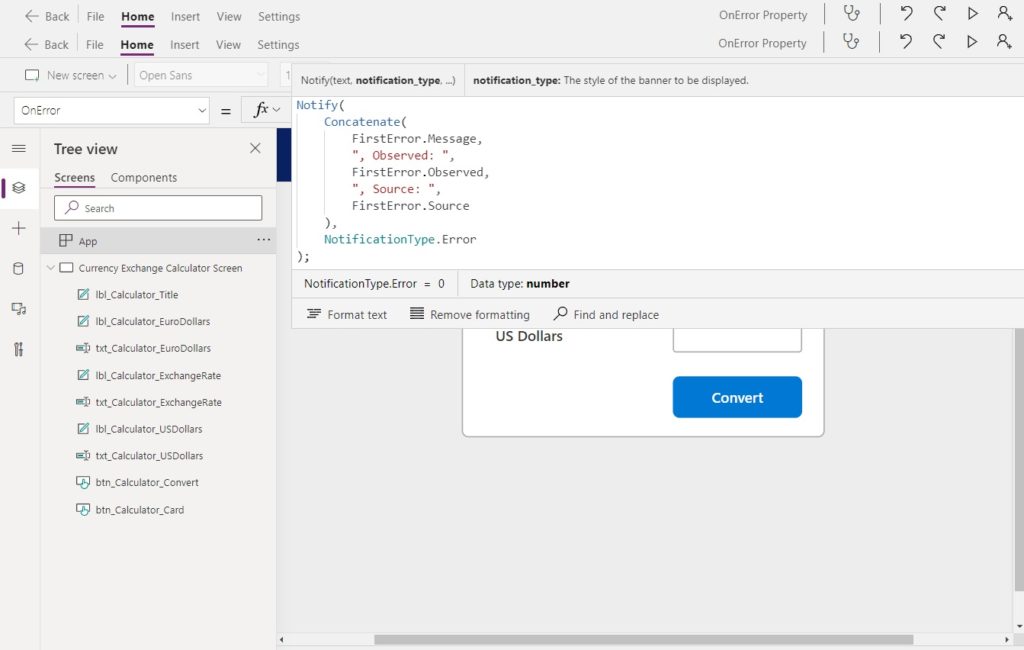
Write this code in the OnError property of the app. It will display a banner at the top of the screen with an error message, the name of the control where the error was observed and the the name of the control where the error initially occurred. FirstError is a special method that can only be used inside the IfError function or the OnError property. For more information on FirstError go read the official documentation.
Notify(
Concatenate(
FirstError.Message,
", Observed: ",
FirstError.Observed,
", Source: ",
FirstError.Source
),
NotificationType.Error
);
Now when we click the Convert button a more helpful error message is shown.
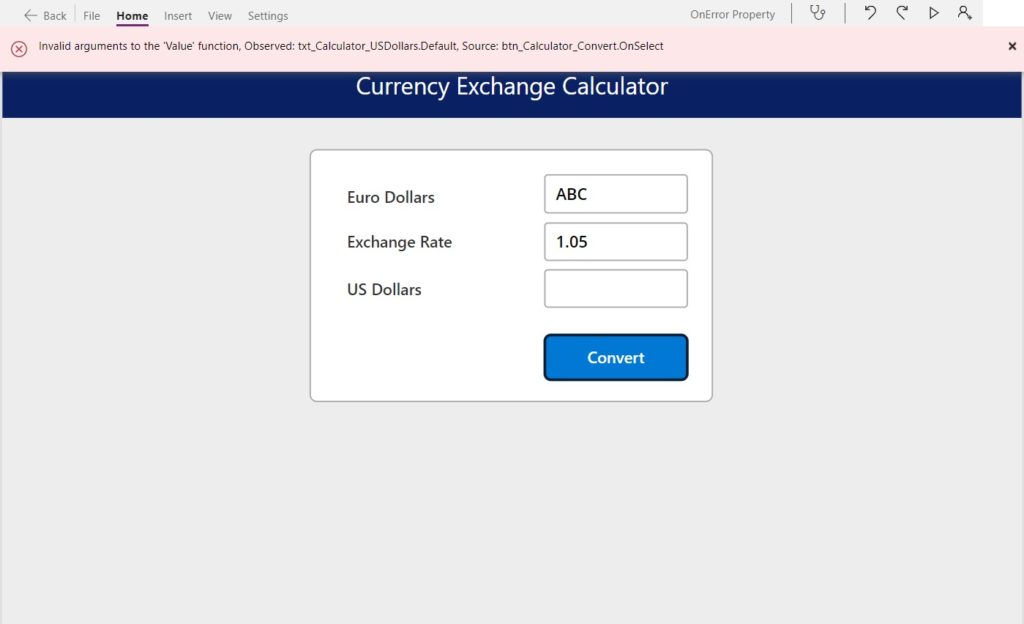
Send Error Details To Power Apps Monitor
Power Apps monitor is a tool developers can use to see all of the activity in a user’s session and debug their apps. Error messages logged by Power Apps monitor do not have much detail by default. We can create our own custom logging with the Trace function to include all the information needed to diagnose and fix a problem
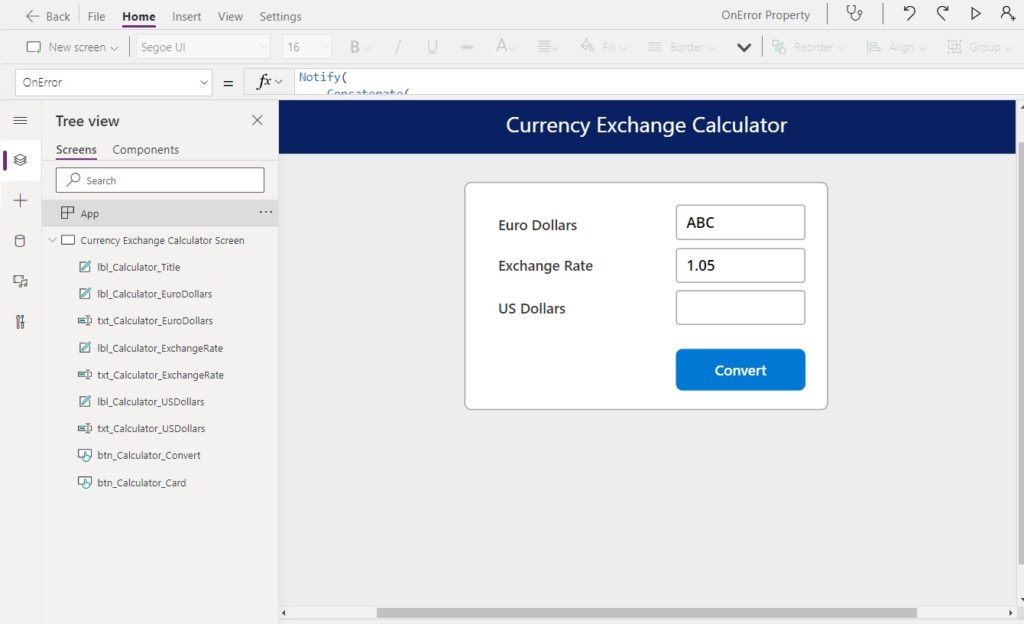
Add the Trace function code to the OnError property of the app. Notice that we are now tracking 6 pieces of information when an error occurs: error kind, error message, the control where the error was observed, the screen the user was on, the control that was the source of the error and user email.
Notify(
Concatenate(
FirstError.Message,
", Observed: ",
FirstError.Observed,
", Source: ",
FirstError.Source
),
NotificationType.Error
);
Trace(
"Unhandled Error",
TraceSeverity.Error,
{
Kind: FirstError.Kind,
Message: FirstError.Message,
Observed: FirstError.Observed,
Screen: App.ActiveScreen.Name,
Source: FirstError.Source,
User: User().Email
}
)
Open Power Apps Monitor from the Advanced Tools menu.
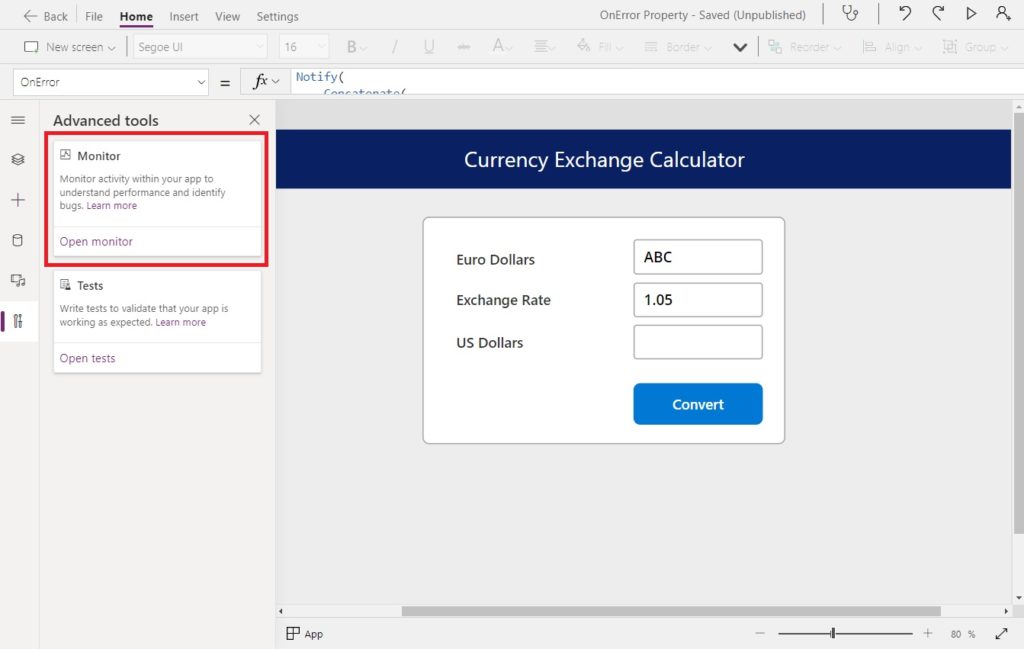
Then generate the error message again and watch it appear in Power Apps monitor. On line 3 of the screenshot below we can see the result of our Trace function.
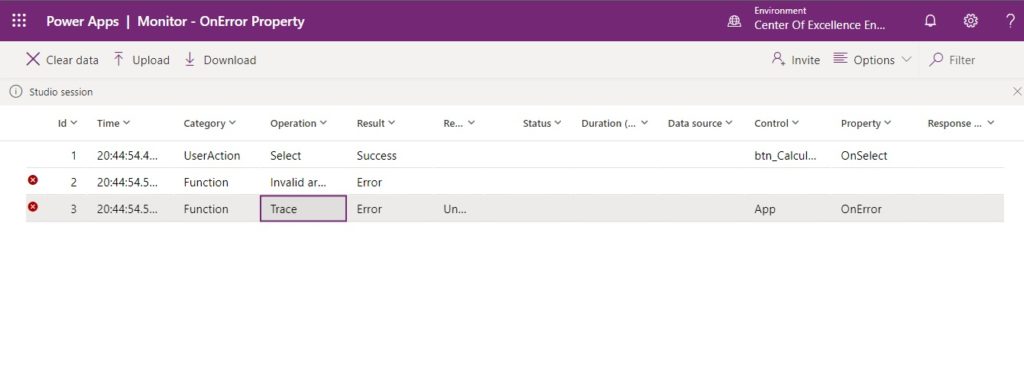
If we drill-into the details of the error we can see the additional information added by the trace.
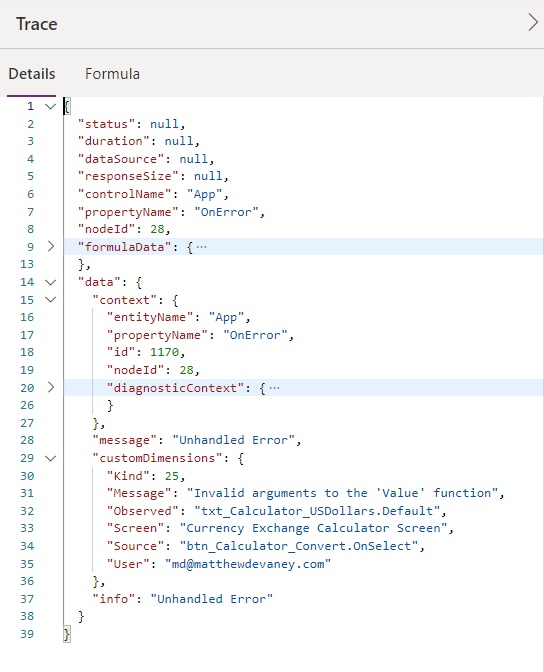
Track Unhandled Power Apps Errors In Application Insights
As a developer, wouldn’t it be useful to know what errors your users are receiving so you can fix them? Most bugs that happen never get reported. But did if we know what errors were happening then we could do something about it and continuously improve the user experience.
Azure Application Insights can be used to log all of the errors captured by Power Apps Trace function. It’s simple to setup and cheap to use – often only a few pennies on your organization’s Azure bill.
Go to portal.azure.com and open the Application Insights service.
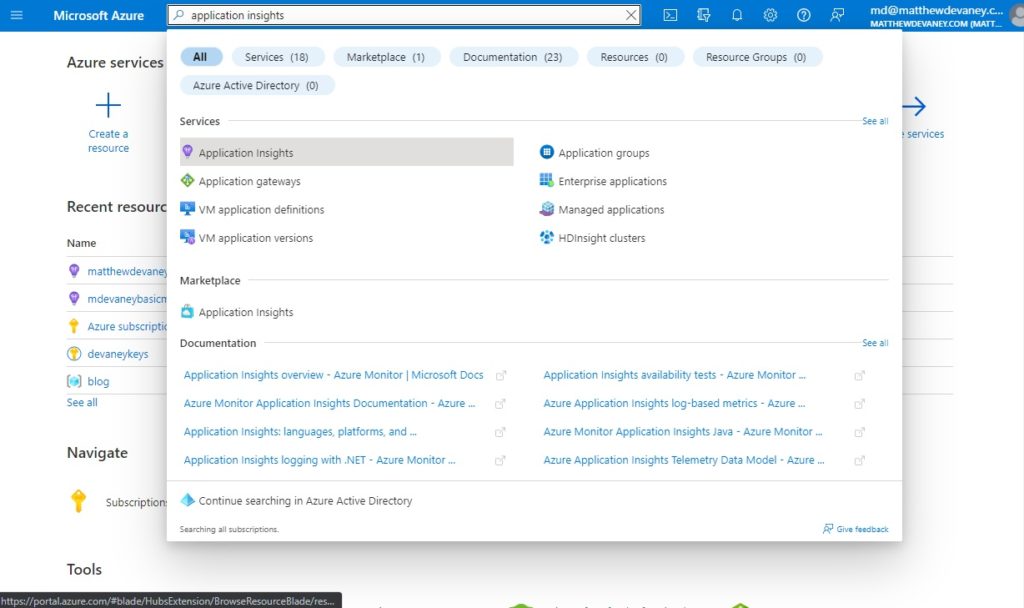
Create a new application.
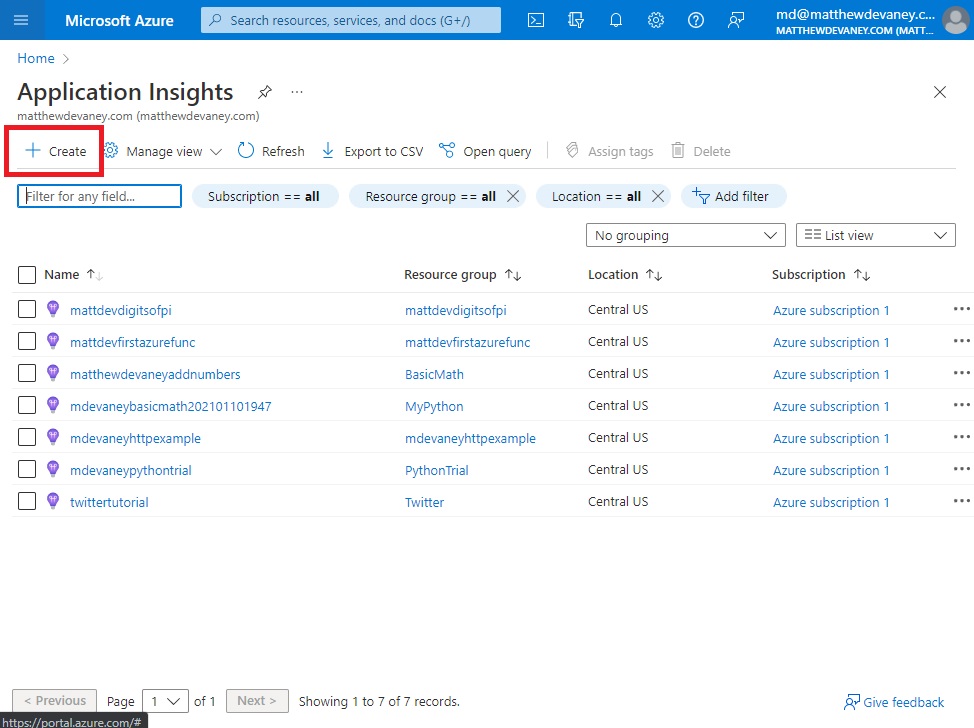
Choose a resource group and give your application a name. I suggest using the name of your app with _PowerApps at the end to make it easily identifiable. Click Review + Create once setup is finished.
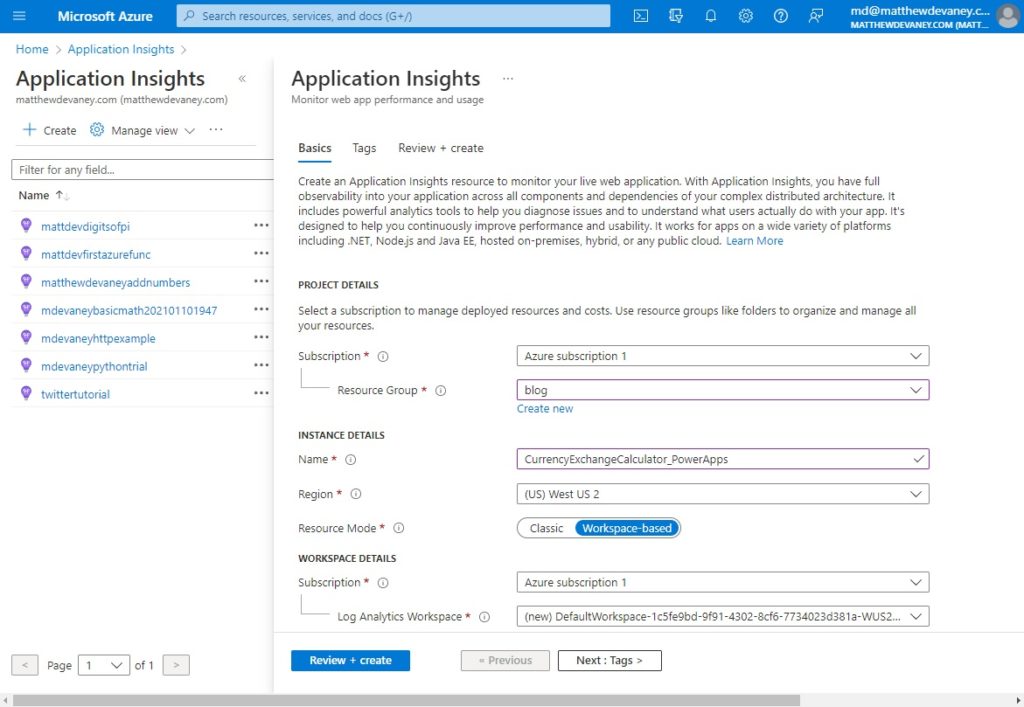
Then click Create on the next screen.
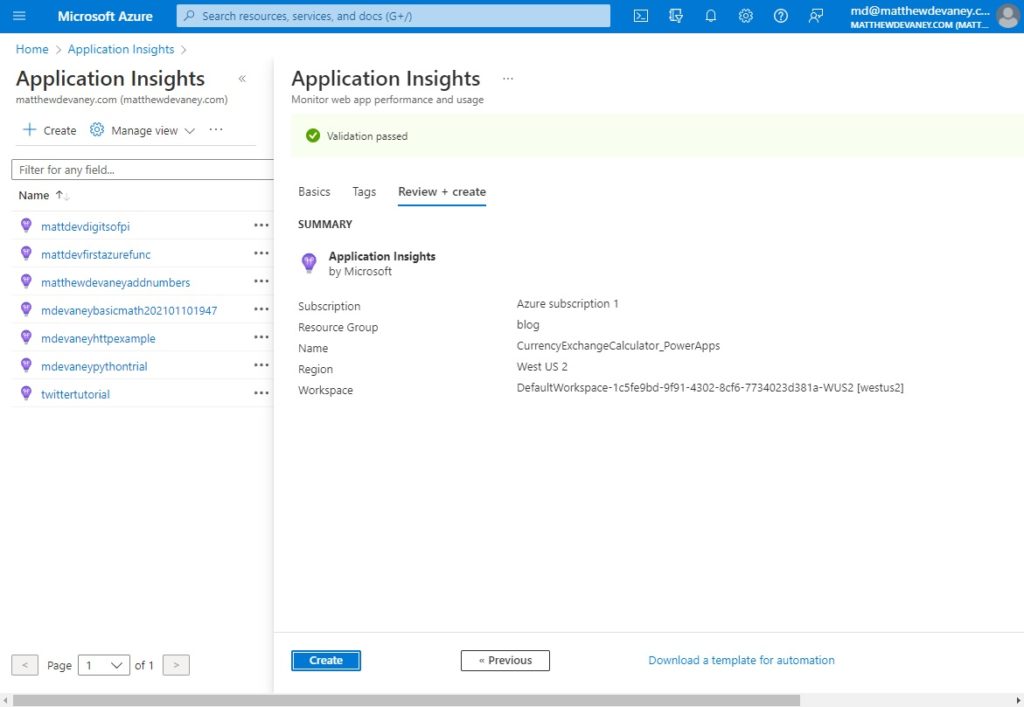
Link The Power Apps App To Application Insights
Now that we’ve create a new app in Application Insights we must link it to our app in Power Apps. Go to the Overview section of the app and copy the Instrumentation Key.
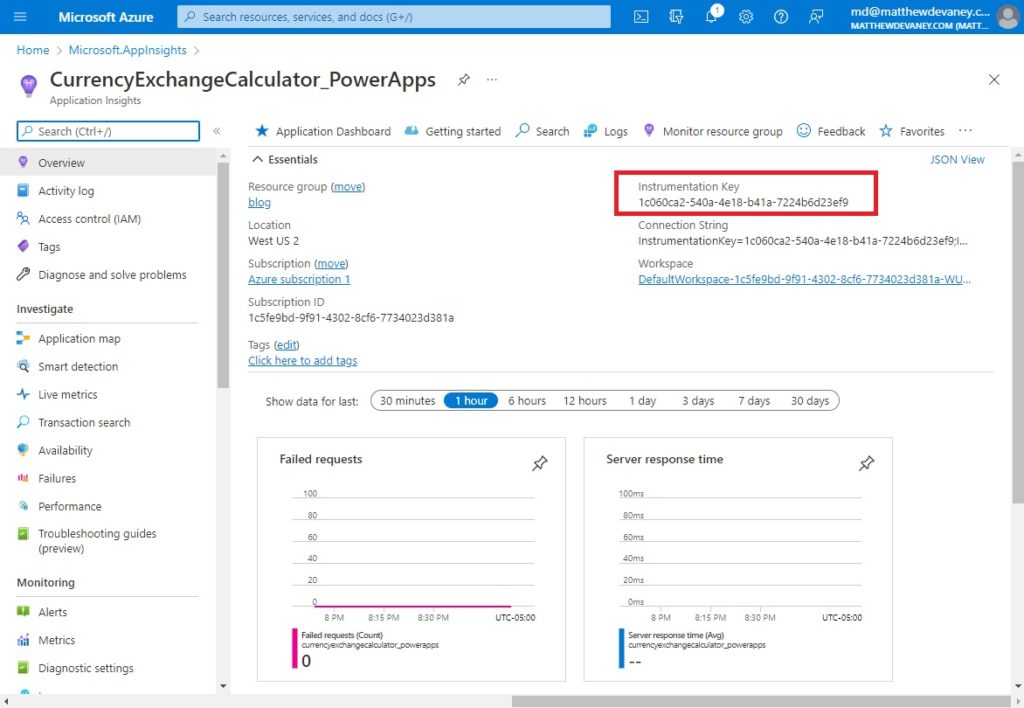
Then go back to Power Apps, select the app object, open the right-menu and paste the Instrumentation Key into the field shown below.
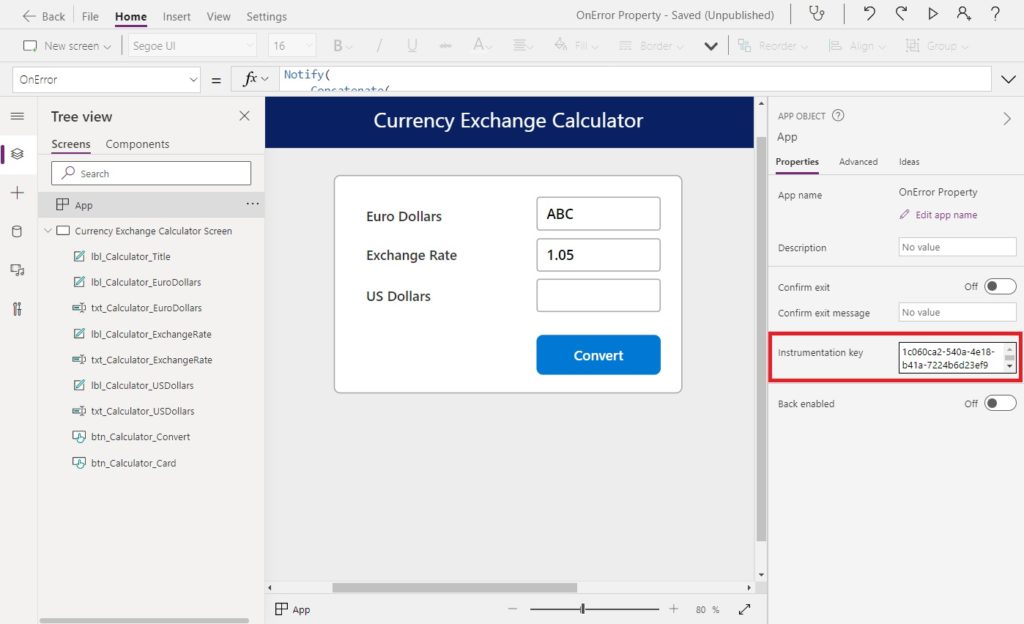
Save and publish the app.
IMPORTANT: the app will not become linked to Application Insights until you save and publish the app |
Get A Report Of All Power Apps Errors In Application Insights
Any errors captured by Power Apps trace function can be viewed in Application Insights. Select Logs from the left-menu of Application Insights.
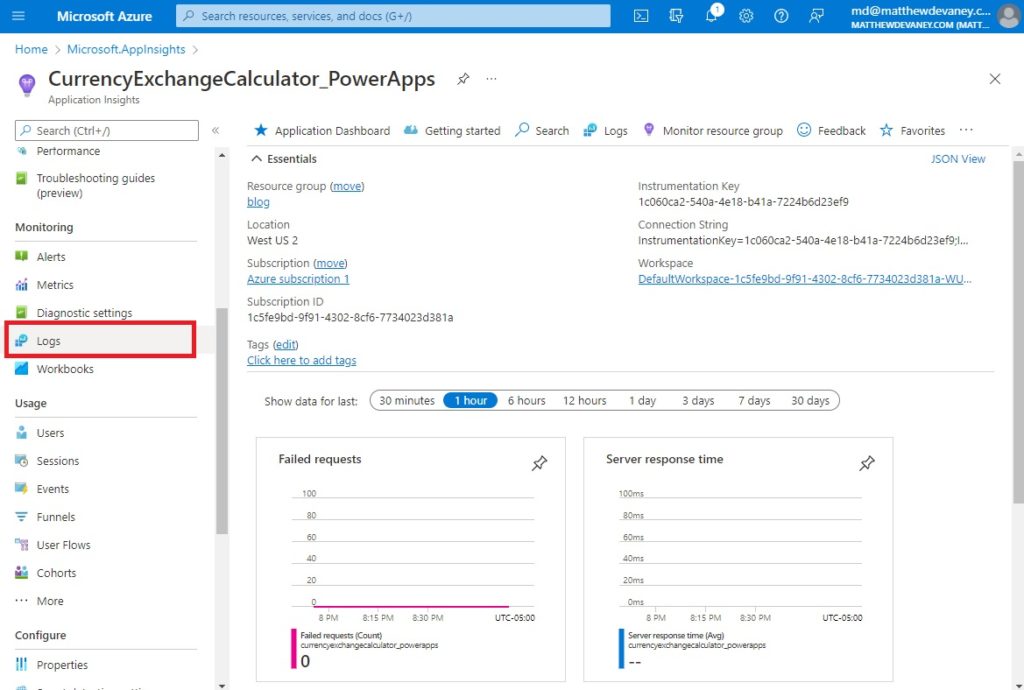
Then write a query to retrieve all of the traces.
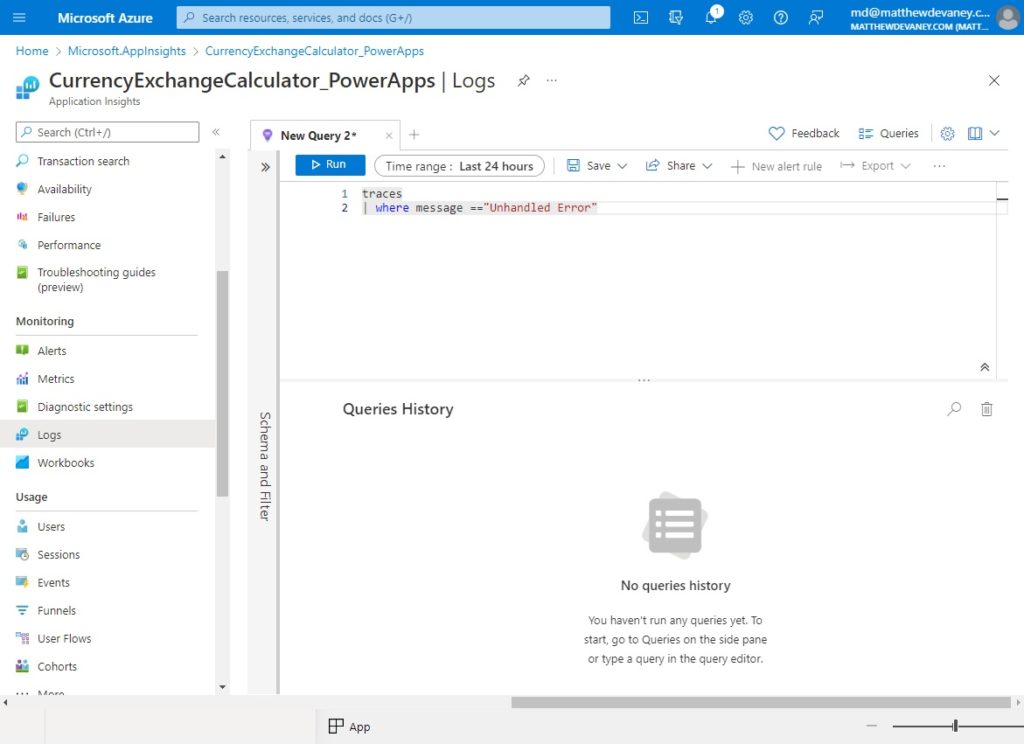
Copy and paste this code into the query editor and click the Run button. Notice that the text “Unhandled Error” is exactly the same as what we placed in our Power Apps trace function.
traces
| where message =="Unhandled Error"
All of the error messages will be displayed in the results field and we can drill-down into them to get detailed reporting. We can export these errors to a CSV file, open them in an Excel spreadsheet or even send them to Power BI!
IMPORTANT: the trace function result will only be logged work while the app is in Play Mode. No traces will be sent during preview mode in Power Apps Studio. |
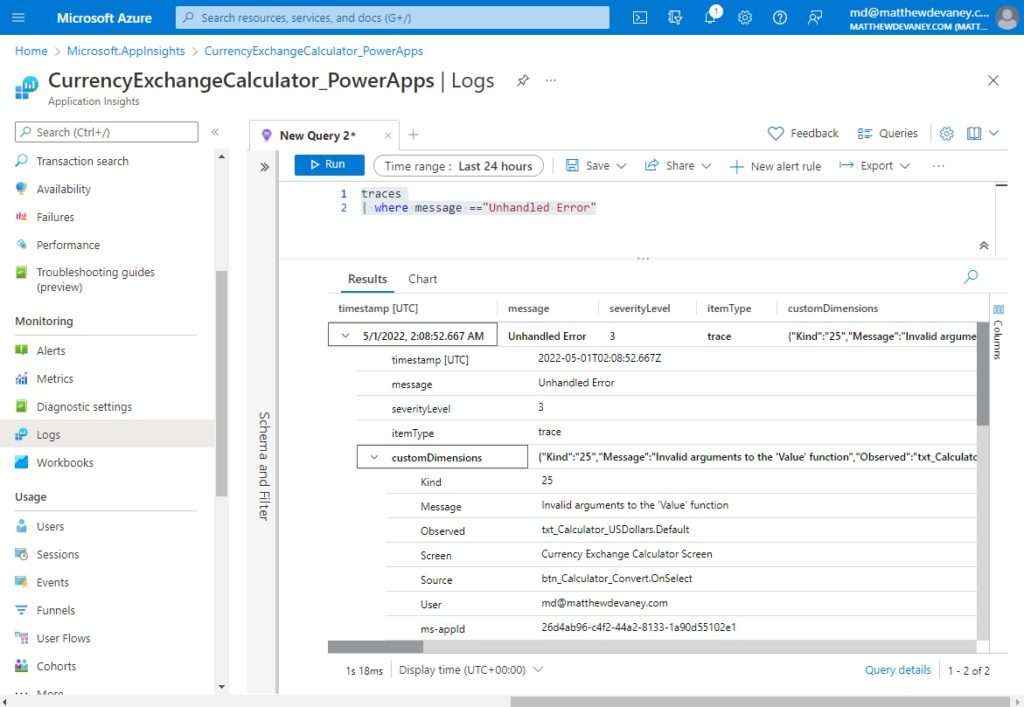
List Of Power Apps Error Kind Codes
As part of our error logging we included the error kind. The error kind is a numeric error code but here is not any documentation on what they mean. I have provided a table of all error kind values and their meanings in the table below.
ErrorKind | Value |
None | 0 |
Sync | 1 |
MissingRequired | 2 |
CreatePermission | 3 |
EditPermissions | 4 |
DeletePermissions | 5 |
Conflict | 6 |
NotFound | 7 |
ConstraintViolated | 8 |
GeneratedValue | 9 |
ReadOnlyValue | 10 |
Validation | 11 |
Unknown | 12 |
Div0 | 13 |
BadLanguageCode | 14 |
BadRegex | 15 |
InvalidFunctionUsage | 16 |
FileNotFound | 17 |
AnalysisError | 18 |
ReadPermission | 19 |
NotSupported | 20 |
InsufficientMemory | 21 |
QuotaExceeded | 22 |
Network | 23 |
Numeric | 24 |
InvalidArgument | 25 |
Internal | 26 |
Did You Enjoy This Article? 😺
Subscribe to get new Power Apps articles sent to your inbox each week for FREE
Questions?
If you have any questions about Power Apps OnError Property: Capture & Log Unexpected Errors please leave a message in the comments section below. You can post using your email address and are not required to create an account to join the discussion.
Hi Matthew ! Nice article. Thanks for sharing.
I don’t know, but “ABC x 1,05” doens’t fire an error message.
Any clue about what I’m doing wrong ?
Oric,
It should be throwing an error… what code did you write in OnError? What about OnSelect of the button? Please share your code here.
I have the same behavior. It seems that the value function just returns Blank instead of throwing an error: https://docs.microsoft.com/en-us/power-apps/maker/canvas-apps/functions/function-value
“If the number is not in a proper format, Value will return blank.”
Button code (sorry, too lazy to rename the fields… ):
Set(varResult, Value(TextInput1.Text)*Value(TextInput1_1.Text));
Reset(TextInput1_2);
Gerry,
I can you please go into File > Settings > Upcoming Features > Experimental and turn on this setting? “Formula level error management”. I believe this is the step I missed in my tutorial.
Works like a charm! Thanks!
Oric,
I added some new instructions to turn on the experimental error handling feature and which authoring version is needed. Can you please check my revisions near the top of the article?
For anyone testing this. Notifications for unhandled errors will only show starting in version 3.21122 of Power Apps Studio.
https://powerusers.microsoft.com/t5/Error-Handling/3-21122-Notifications-for-unhandled-errors/td-p/1386911
Derek,
Good point. The automated notifications were introduced a few months ago along with OnError. Older apps will need to be republished under a higher authoring version to see the changes.
Thank you for this! I’ve been struggling with one particular app – an error message flashes on the screen but I haven’t been able to pinpoint what was going on and it hasn’t stopped anyone from continuing on with what they were doing. I’ve used your instructions to fill out the Error notification message AND to send myself an email with all these details when the app has an error since people often don’t let me know. I now know what’s wrong and can fix it 🙂 I’m going to bring up the other app you mentioned to see if our organization will purchase it, but even if they don’t, this has been so helpful!
Eileen,
Yes, definitely ask your organization to adopt Azure Application Insights. It’s dead simple to setup and they’ll hardly notice the cost on their Azure bill because it’s just transmitting a row of text from the app.
If you start using App Insights please make a follow up post. I want to know how it improved things for you.
Great article, thanks for sharing. I have one question about “List Of Power Apps Error Kind Codes”. You mentioned that the enumeration values are undocumented. I am interested to know how you found out which error code was which?
Hello Ali,
When I typed in the keyword ErrorKind the autocomplete showed me the list of available options. Then I typed out each one to see the number. It was boring but I got the info I wanted.
Thank you, Matthew.
Error kind #26 exists but is not listed
Gergely,
Thank you. I’ve added Internal error to the list.
Broken Link
Enable The Formula-Level Error Management Setting
https://www.matthewdevaney.com/power-apps-onerror-property-capture-log-unexpected-errors/Enable-The-Formula-Level-Error-Management-Setting
Mr. Link,
Thanks for reporting the broken link. You are a saint!
Hi Matthew, awesome article, keep it up, buddy.
I hope you can give me a hand on 2 issues that I have no clue what’s wrong with them.
The Patch() below generates an error called “Network error when using Patch function: Field ‘Title’ is required” even though, as you can tell, I am indicating the Title in the Patch()
And the most critical one
I wrote in my App.OnError the below Patch().
Most of the unexpected errors are being saved, however. when the end users try to run a flow in Power Automate, sometimes it generates an error, but the title is blank, so I have no idea what the problem is.
I hope I made myself clear
Johany,
Hmmmm, can you please double check that AdtProcess.Title is loaded with a value? It would be my first guess. That you’ve coded it properly but the variable has no value.
What is your datasource here by the way. Is it SharePoint? Also, have you tried debugging with Power Apps monitor? You might be able to get some more info…
Hi Matthew.
Yes AdtProcess.Title is not blank…
I found you suggested to someone in the community to clear the cache, I did it and it worked for a couple of days, I just noticed that issue came back.
Yes, my DB is Sharepoint
How can we customize the error message we get when a user is not having access to the share point list used as datasource in powerapps:
For example the error I get is,”error while retriving the data from network”, how can i change into a meaningful message of my choice
Thank you, from the bottom of my heart, for writing this. I spent multiple hours trying to figure out where an error on an unknown text label amongst 100+ labels was coming from and was losing my mind because it wasn’t getting sent to the monitor and I had no idea which label it was… this is post is a miracle. 🙃
Christine,
Oh my. A 100+ field form sounds like a challenge to diagnose the issue. I am glad my post could help in some way 🙂