Table Of Contents:
• Enable Formula-Level Error Management
• Patch Function Error-Handling
• Power Apps Forms Error-Handling
• Power Automate Flow Error-Handling
• IfError Function
• Handling Unexpected Errors
Enable Formula-Level Error Management
Open Power Apps advanced settings and turn on formula-level error management. This setting enables use of the IfError function, the IsError function and the app’s OnError property.
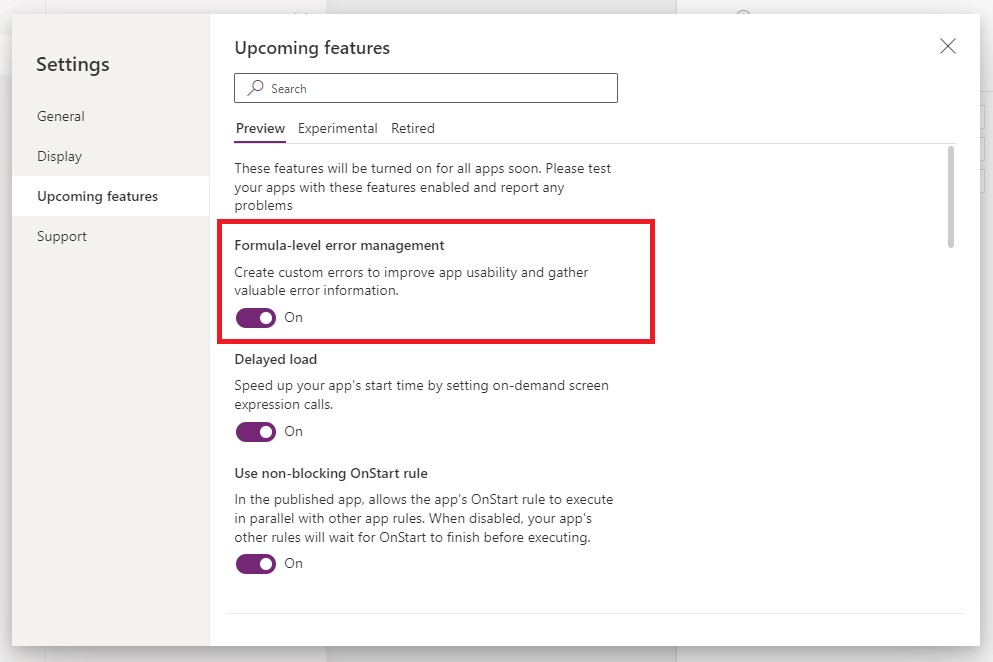
Patch Function Error-Handling
Check for errors anytime data is written to a datasource with the Patch function or Collect Function. Even if the submitted record(s) are valid, good connectivity and the correct user permissions cannot be assumed. This is not necessary for local collections stored in memory.
// Create a new invoice
If(
IsError(
Patch(
'Invoices',
Defaults('Invoices'),
{
CustomerNumber: "C0001023",
InvoiceDate: Date(2022, 6, 13)
PaymentTerms: "Cash On Delivery",
TotalAmount: 13423.75
}
)
),
// On failure
Notify(
"Error: the invoice could not be created",
NotificationType.Error
),
// On success
Navigate('Success Screen')
)
Power Apps Forms Error-Handling
Write any code should be executed after a Power Apps form is submitted in its OnSuccess and OnFailure properties. If form submission is successful, use the OnSuccess property to control what happens next. Otherwise, use the OnFailure property to display an error message that tells the user what went wrong.
// OnSelect property of the form's submit button
SubmitForm(frm_Invoice);
// OnSuccess property of the form
Navigate('Success Screen');
// OnFailure property of the form
Notify(
"Error: the invoice could not be created",
NotificationType.Error
);
Do not write any code after the SubmitForm function used to submit the form. If form submission fails Power Apps will still move onto the next line of code. This can result in loss of data.
// OnSelect property of the form's submit button
SubmitForm(frm_Invoice);
Navigate('Success Screen');
Power Automate Flow Error-Handling
When a Power Automate flow is triggered from Power Apps its response must be checked for errors. Flows can fail due to poor connectivity. They can also return a failure response or a result with the incorrect schema. If Power Apps does not know the flow failed it will continue as normal.
// Get customer invoices
If(
IsError(
GetAllCustomerInvoices.Run("C0001023")
),
// On failure
Notify(
"Error: could not retrieve customer invoices",
NotificationType.Error
),
// On success
Navigate('Success Screen')
)
IfError Function
Use the IfError function to handle calculations that require a different value when an error occurs. In this example, if gblTasksTotal equals 0 the IfError function will return 0 instead of throwing a “divide by zero” error.
// calculate the percentage of tasks completed
IfError(
gblTasksCompleted/gblTasksTotal,
0
)
Handling Unexpected Errors
An app’s OnError property is triggered when an unexpected error occurs. An unexpected error is any error that is not handled using the IfError or IsError function.
Use this code to quickly locate the source of unexpected errors and fix them. Do not leave this on in a production app since the error message is helpful for a developer but is confusing to a user. If you want to see unexpected errors in a production app use the Trace function and Azure Application insights to silently log the errors.
// unexpected error notification message
Notify(
Concatenate(
"Error: ",
FirstError.Message,
"Kind: ",
FirstError.Kind,
", Observed: ",
FirstError.Observed,
", Source: ",
FirstError.Source
),
NotificationType.Information
);
Did You Enjoy This Article? 😺
Subscribe to get new Power Apps articles sent to your inbox each week for FREE
Questions?
If you have any questions about Power Apps Error Handling Guidelines please leave a message in the comments section below. You can post using your email address and are not required to create an account to join the discussion.
Thankyou so much for this solution. It helped me a lot. I was searching this from very long
Deepika,
You’re welcome. I am looking forward to presenting on Power Apps & Power Automate error-handling at Microsoft Power Platform Conference 2023 🙂
This is great as always Matthew! I am looking to implement functionality such that whenever an app user encounters an error on production app, an error notification should be sent to the developer immediately over email & logged in a sharepoint list that has all error logs. For example, let’s say the developer has changed data types of 2 columns in the underlying datasource of the prod app, the app user will get some errors in the app due to data type mismatch. These errors need to be notified to the developer and logged in the sharepoint error logs list. How can the above be achieved using PowerApps & PowerAutomate (only to send email notifications and update the errorlogs sharepoint list)? Any ideas and solution to the above is highly appreciated
Saurabh,
If you want Power Apps error-logging there is no better way to do so than by using Application Insights. You will get a queryable log that shows everything!
https://www.matthewdevaney.com/power-apps-onerror-property-capture-log-unexpected-errors/#Get-A-Report-Of-All-Power-Apps-Errors-In-Application-Insights
For Power Automate error notifications check this article:
https://www.matthewdevaney.com/3-power-automate-error-handling-patterns-you-must-know/
How can I prevent submission of a form when there is an incorrect format typed in a field? For example, if an error message appears to the user under a form card, the user should not be able to submit the form. Maybe I am going about it the wrong way. Thank you.
Karim,
Can you give a sample format?
Great site! I just love you clear & concise explanations.
How would you implement error handling for a patch statement for which the result is returned to a variable? Eg. Set(VariableName, Patch(Datasource, BaseRecord, ChangeRecord))
Jottie,
Check out my guide on the PATCH function. It has the answer to your question:
https://www.matthewdevaney.com/7-ways-to-use-the-patch-function-in-power-apps-cheat-sheet/#3.-Get-The-Result-Of-The-Patch-Function
Matthew, can you elaborate more here. Not clear. Like Jottie, I am setting a variable equal to the Patch syntax. That variable is used as the default in a gallery so when a new record is patched, the gallery defaults to this record.
But I can not figure how to use the Iserror, iferror, errors table, etc to notify the user of an issue.
Scott,
Here’s a sample…
Set(
gblInvoiceRecord,
Patch(
‘Invoices’,
Defaults(‘Invoices’),
{
CustomerNumber: “C0001023”,
InvoiceDate: Date(2022, 6, 13)
PaymentTerms: “Cash On Delivery”,
TotalAmount: 13423.75
}
)
);
// Create a new invoice
If(
IsError(gblInvoiceRecord),
// On failure
Notify(
“Error: the invoice could not be created”,
NotificationType.Error
),
// On success
Navigate(‘Success Screen’)
)
Hello!
How would multiple IsError()’s be handled in a Concurrent() function?
Example:
Concurrent(
Patch(MY_DATA_SOURCE,
Defaults(MY_DATA_SOURCE),
colTest
),
TestFlow1.Run(colFlow1),
ForAll(colPhotos,
TestFlow2.Run(ThisRecord.img)
)
).
When I tried to do it in a single IsError() function, it all appears as a single function and Concurrent() error’s out (“expected 2 or more functions…”).
Thanks!
May i ask, what happen using iferror function it will return blank()? Can it implement in patch record?
Aliah,
I do not suggest using IfError to check the result of a PATCH. Instead, store the PATCH result in a variable and test it with the ISERROR function.
Hey Matt, can I ask why you don’t suggest using IfError to manage patch errors when Microsoft use it in their documentation here? https://learn.microsoft.com/en-us/power-platform/power-fx/reference/function-iferror#iferror. I’m trying to work out the benefits of using If and IsError together when IfError seems to do the same thing?
Great Blog post
I hate to turn on formula level error management as long as it is in Preview mode. You never know if Microsoft will pull some whizbang and cause code which was working with this feature on suddenly start to bomb. Any idea when they’re going to get this moved into production?
If It’s not Experimental it is going to be released. Further, that feature has to be implemented. Microsoft has no choice as its crucial functionality.
I agree that it will be released and is critical functionality.
how i can achieve display the error message from power automate to power datatable
Muthuselvi,
There is a new Dataverse table called “Flow Runs”. Once that feature gets rolled out I think you’ll be able to see your flow history there and get the error message.
How can we customize the error message we get when a user is not having access to the share point list used as datasource in powerapps:
For example the error I get is,”error while retriving the data from network”, how can i change into a meaningful message of my choice
Anon,
Use the OnError property of the app to check the Kind property of the error. Then use an IF statement to control which message is displayed within the notify function.
I can’t use the last code in my app, it doesn’t see to recognize the FirstError object? it reads like the code would just work…I can use IfError and IsError functions
Pablo,
You must use this code in the App’s OnError property.
ah, ok, thank you for the clarification Matthew
Have a great week
The Respond to Power Apps step within a flow doesn’t work if the previous step takes longer than two minutes to complete. That’s why I’m wondering if If a flow takes longer than two minutes to run, will IsError still work right?
Hi Matthew, does this work for you? Looks like Office365Users.ManagerV2 cannot be captured by IfError – or If(IsError(…), …) for that matter. Is there any other way to check if a user has a manager?
Set(
ManagerOfSessionUser,
IfError(
Office365Users.ManagerV2(User().Email),
Blank()
)
)
error code 8 – requested operation is invalid. Unable to pinpoint where the problem is. Same Patch code works during Edit save. Defaults gives me this error on one of the list. A similar list with similar patch code works fine in both cases, defaults and Edit.