$-Strings: A Better Way To Concatenate Text Strings In Power Apps
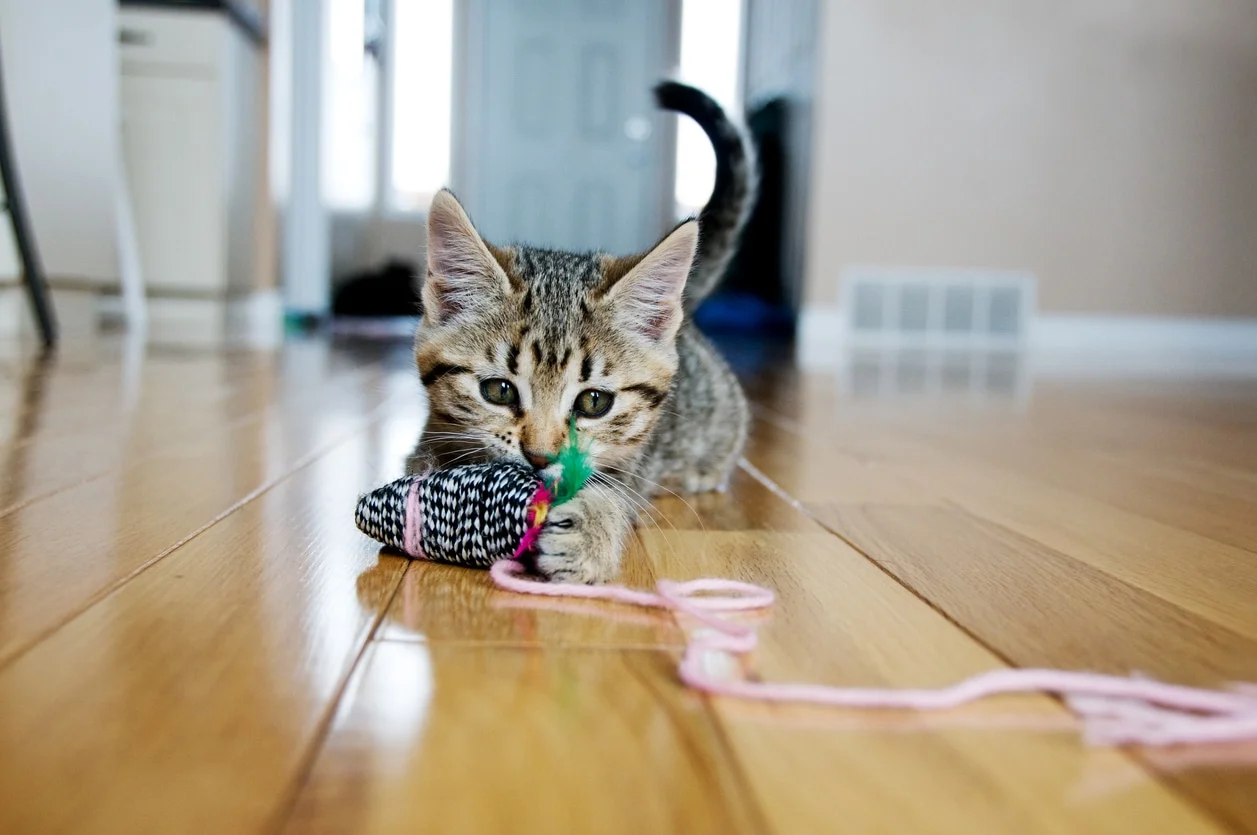
An awesome new way to concatenate text strings in Power Apps has arrived with $-Strings. Placing the $ symbol in front of any text string now defines it as an $-String. Then you can write any function, expression of variable inside a within a pair of curly braces { } inside the text string. I love this syntax and I can’t wait to share it with the world. In this article I will show you how to use $-Strings to concatenate a text string in Power Apps.
Table Of Contents
• The New Way To Concatenate Text With $-Strings
• $-String Usage Examples
⚬ Referencing Controls
⚬ Reading Variable Values
⚬ Math Expressions
⚬ Concat Function
⚬ Error Handling
• Other $-String Details
⚬ Curly Braces
⚬ Quotation Marks
The “Old Way” To Concatenate Text Strings In Power Apps
A common thing we want to do in Power Apps is show the app user a welcome message with their name and the current date.
Hello, Matthew Devaney. The current date is 4/10/2022.
The message is a made up of two elements: text used to define the standard greeting users will see and functions used to obtain their name and the current date. To display these elements as a single message we can use the CONCATENATE function to join them together. Each section of text or function is a supplied as a new argument separated by a comma. It works, but the code itself is somewhat verbose and not very readable.
Concatenate(
"Hello my name is",
User().FullName,
". The current date is",
Today(),
"."
)
Another way to join multiple text strings and functions together is with & operator. Instead of writing a CONCATENATE function we simply write an & symbol between each section just like Microsoft Excel. This more readable during a code review but I find it a pain to type the extra & symbol and quotation mark between each segement.
"Hello my name is" & User().FullName &". The current date is" & Today() & "."
The New Way To Concatenate Text With $ Strings
My preferred way to concatenate text strings in Power Apps is by using the new $-String syntax. To do this we put a dollar sign ($) in front the text string’s quotation marks and use curly braces {} to denote the parts of it which are expressions – functions, formulas or variables that might change. The official term for this technique is string interpolation and it is found in many other programming languages.
$"Hello {User().FullName}. The current date is {Today()}."
I enjoy $-Strings because they require the least amount of syntax to write and my code is easily readable when reviewing it.
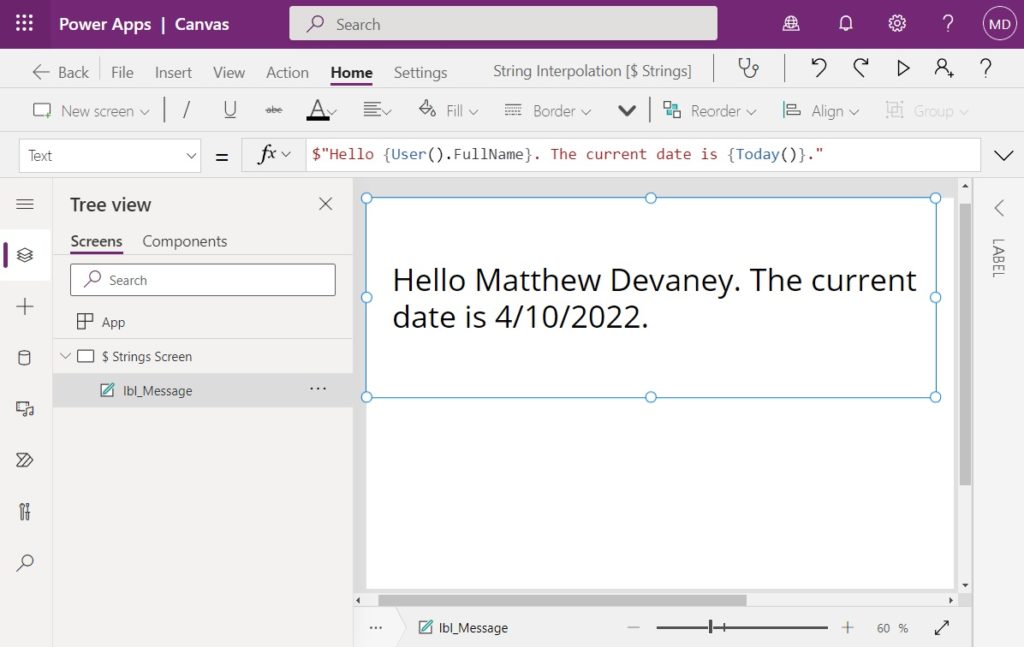
$ String Usage Examples
Let’s take a look at few more examples of how we can use $-Strings to concatenate text in Power Apps.
Referencing Controls
We can join the text from a text input and selected date from a date picker to other text strings like this:
$"Hello {txt_Username.Text}. The current date is {dte_CurrentDate.SelectedDate}."
The result looks like this:
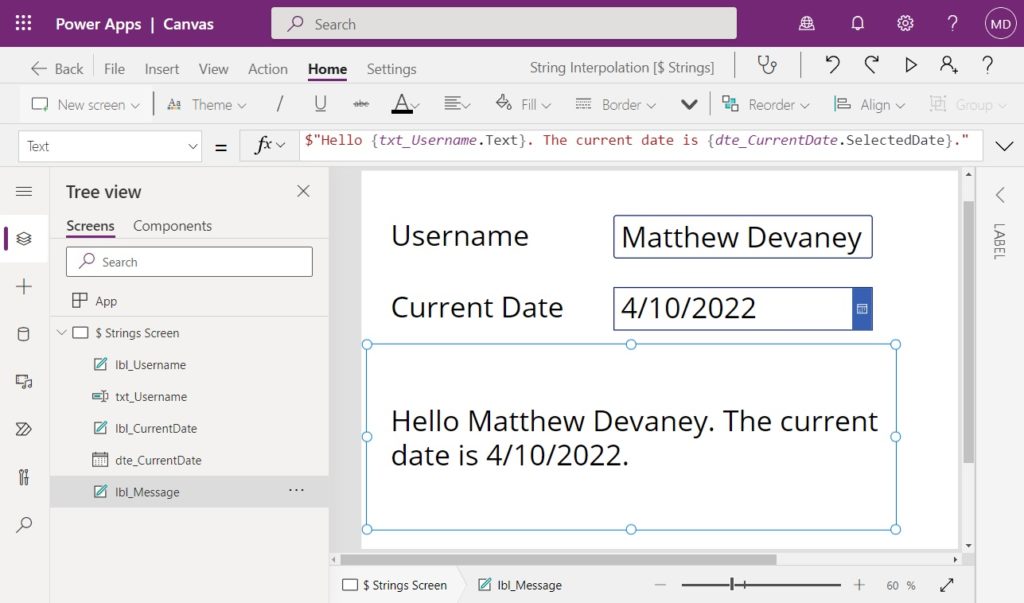
Reading Variable Values
We could also choose to store the result some functions in variables and use those variables inside the $-String.
// set variables elsewhere in the code
Set(varUsername, User().FullName);
Set(varToday, Today());
// use this value in the Text property of a label
$"Hello {varUsername}. The current date is {varToday}."
Math Expressions
Writing mathematical expressions inside of $-strings is an interesting use case. Anything inside the curly braces can be a formula so we can directly write 4 * 3 inside the text string to find the result of 12.
$"{4} multiplied by {3} equals {4*3}"
Here’s a similar example of how we could multiply two values found within text inputs and show the result
$"{txt_Number1.Text} multiplied by {txt_Number2.Text} equals {Value(txt_Number1.Text) * Value(txt_Number2.Text)}"
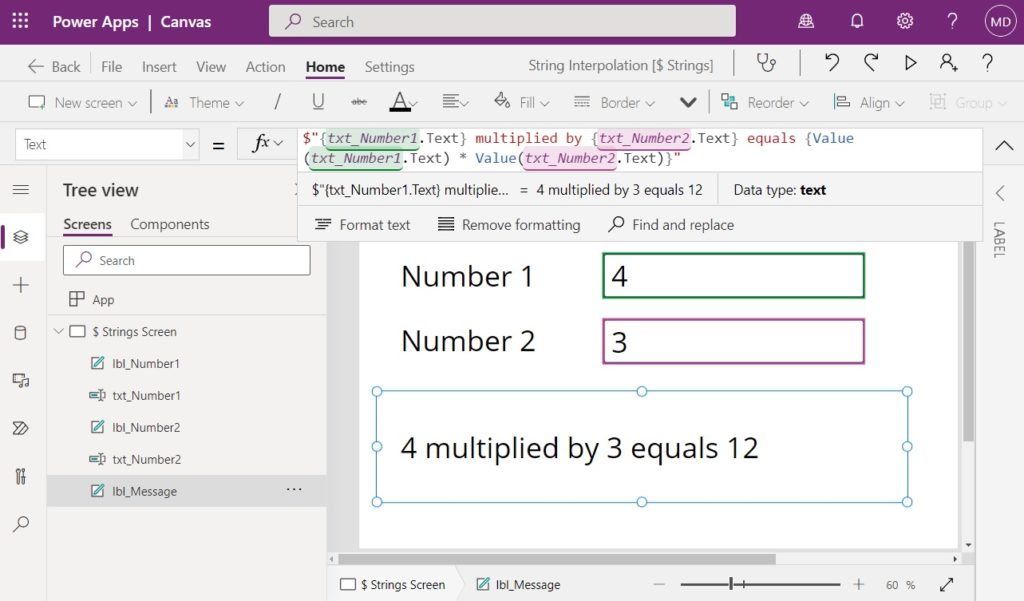
Concat Function
The $-String is quite valuable when using the CONCAT function. CONCAT joins the result of a formula applied to all records in a table and outputs a single text string. In this example I have created a table holding the numbers 1-through-5 and want to know their squares.
Concat(Sequence(5), $"{Value} squared equals {Value * Value}.{Char(13)}")
The resulting text string looks this:
1 squared equals 1
2 squared equals 4
3 squared equals 9
4 squared equals 16
5 squared equals 25
Error Handling
One final use case for $-Strings is error-handling. We can place the following code within the OnError property of an app to show the developer a helpful error message.
Notify(
$"Error was observed at {Error.Observed}: {Error.Message}",
NotificationType.Error
)
The resulting error message would look something this. This specific error message is saying the app cannot perform division by zero and the value entered in the text input txt_Number is causing the problem.
Error was observed at txt_Number: Cannot divide by zero
Other $-String Details
When concatenating text with Power Apps $-String notation you might have the following difficulties.
Curly Braces
Curly braces are rarely needed inside text strings but what if we wanted to display a message like this?
This is how you write curly braces {} in Power Apps $-Strings.
We can show curly braces inside of an $-String by placing one pair of curly braces inside another.
$"This is how you write curly braces {{}} in Power Apps $-Strings."
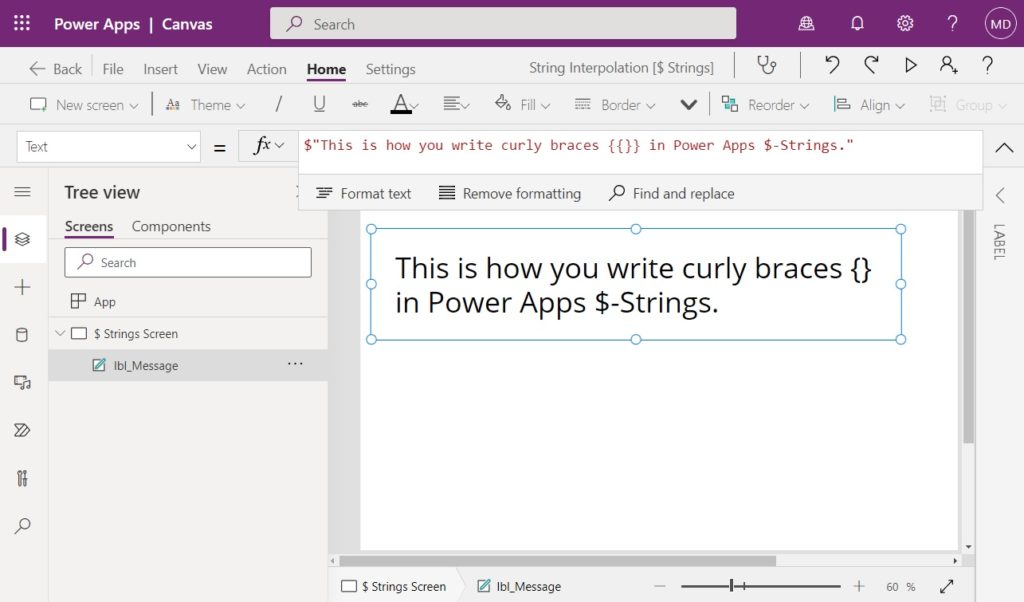
What if a word needed to be shown within curly braces?
I can also write words inside curly braces {like this}
Again, we simply have to write two-pairs of curly braces with the word in the middle.
$"I can also write words inside curly braces {{like this}}"
Quotation Marks
Quotation marks are another challenge because they are used to identify text strings. What if we wanted to write the sentence below?
The English word "Hello" has the same meaning as the French word "Bonjour"
By writing 3 sets of quotation marks we can tell Power Apps to display a single-set of quotation marks surrounding the words “Hello” and “Bonjour”.
$"The English word {"""Hello"""} has the same meaning as the French word {"""Bonjour"""}"
Did You Enjoy This Article? 😺
Subscribe to get new Power Apps articles sent to your inbox each week for FREE
Questions?
If you have any questions about $-Strings: A Better Way To Concatenate Text Strings In Power Apps please leave a message in the comments section below. You can post using your email address and are not required to create an account to join the discussion.
Hi, are there any usecases where old way would not work?
Thanks
Radek,
No, the old way will still work because $-Strings have a $ sign in front of them.
Enjoyed your article Matt. Please notice a small mistake in Set(varToday); line.
Vipin,
I will fix it immediately. Thank you for taking the time to write me and let me know about the error. I am thankful to have readers such as yourself who can help me do quality control on the blog 🙂
Matt, I can’t seem to get this to work – I’ve tried as simple as: –
And still get an error. Am I missing something? Is the dollar character different according to regions?
David,
Did you make sure you are on the latest authoring version described at the top of the article? I had to set the new authoring version manually.
That’s great, thanks!
Do you have an examples of how you would conditionally insert extra text into the middle of one of these strings? For example I could currently do: –
$”String {If( true, “ent”)} something”
I am getting an error trying to do this. In my case I am adding a dash and then the value of another text input. I am not sure I am doing it right though as I am getting the “Expressions which appear inside an interpolated string must evaluate to a Text value or to a compatible type”
So this:
$”##{tiCountry.Text} {tiCity.Text} – {tiEquipmentName.Text}”
Needs to be this:
$”##{tiCountry.Text} {tiCity.Text}{If(cbMultipleBuildings.Value, “-” & tibuildingidentifier.Text)} – {tiEquipmentName.Text}”
Very cool article, I didn’t know about the $ sign before
Sultan,
It’s a brand new feature. You are hearing about it on my blog before any official announcement from Microsoft.
OK, but how do you pronounce “$-String”?
George,
Dollar-string? Money-string? Washington-string (US)? I’m going with dollar strings personally, LOL.
I’m going with “String-string”, but that’s because I’m old.
We are calling it String Interpolation, the same term used by C#. And if you Bing the definition of interpolation (which I had to do), it fits pretty well: “the insertion of something of a different nature into something else.”
Nice,
Time to update my apps.
What about HTML texts, how it works?
Gus,
$-Strings will not work in HTML text because the curly braces are not a part of HTML.
Tx,
I have prove the concept and works prefectly!
Everything comes a mess when published.
For some reason, PowerApps did not publish the App correctly, even if during editing, everything looks correct.
Another great write up. Thanks for taking the time.
$”Hi Matthew, love it sooo much 🙂 Especially when putting together URLs or HTML from data in the app that is so much easier to read and proof. Thanks for sharing.{so_happySmilingFace}”
Hi Matt, after reading your article I’ve given it a try and I have some strange things happening once I close the app. All works fine but when I save and close the app and come back to it later all of my $ have been automatically changed to the word Concatenate and my whole app is covered in errors!
E.g my code was: Launch($”tel:{Label1_2.Text}”)
After saving, closing & re-opening it no reads: Launch(Concatenatetel:{Label1_2.Text}”)
Any ideas why?
Katherine,
Wait, what?!? It replaces the $ with CONCATENATE? That is not supposed to happen. It is clearly a bug in the programming. Do you know how to re-create this bug in a blank app? What authoring version are you using? If you can tell me how to also get this error I will report it to Microsoft so it gets fixed.
I have had the same problem that Kathrine have.
By the way, I changed some codes in my app with de $-Strng approach, everything looked great, but when open the app was obligated to return to old way, cause code was chaged automatically to what exactly Kathrine described.
I’m on Authoring version 3.22041.27 btw
Rob,
I’m using the same version as you but I cannot replicate the issue. What formula are you using inside the $-String?
I replaced:
Claims: Concatenate(“i:0#.f|membership|”, currentUser.Email),
with
Claims: $”i:0#.f|membership| {currentUser.Email}”
I then saved, Published and exited. When I restart the code then shows;
Claims: Concatenatei:0#.f|membership| {currentUser.Email}” ,
and is broken.
After correcting back to the original all is well.
However… I created a new app using your example
// set variables elsewhere in the code
Set(varUsername, User().FullName);
Set(varToday, Today());
// use this value in the Text property of a label
$”Hello {varUsername}. The current date is {varToday}.”
I then created another label with
Concatenate(“Hello ” & varUsername , “. The current date is ” & varToday)
Saved, Published and reloaded both labels stayed the same.
I then changed the Concat to the $ string then etc… and it remained intact!
So I’m baffled! Perhaps it may have something to do with the fact that the app I changed pre-dates the update? The fact remains that I can’t use the $ String in an existing app….
I should point out that the $ string change worked fine before saving & publishing & reloading on the old app
There was unfortunately a bug in the initial release of this feature that causes this behavior in some scenarios. It has been fixed in 3.22051 which will be available soon.
Hi Matt,
Thanks for the article – that’s very cool!
However, I can report the same issue as others. $” (dollar sign and the initial quotation mark) get replaced by the word Concatenate upon saving my app.
I’m on version 3.22041.27, but my app predates it.
I’ve initially changed some of my HTML Text fields (it worked wonderfully) only for them to be broken when I saved and re-opened the app. I also tested it in the same app on a plain Label field with a very simple string and observed the same behaviour.
There was unfortunately a bug in the initial release of this feature that causes this behavior in some scenarios. It has been fixed in 3.22051 which will be available soon.
Thanks, Greg! Appreciate you taking the time to inform everyone individually. Looking forward to the new release.
Hi Matthew,
So sorry I’ve only just seen your response, I am on authoring version 3.22042.8 and it was a brand new app that I created the same day I made my first comment.
I can confirm this behaviour. I also made some changes and came back to the same experience Katherine had.
Plus: The German version of make.powerapps.com uses (don’t ask me why) semicolons when the English version uses commas in the code. When I saved my app and opened it again not only had the editor replaced the $ with ‘Concatenate’ but it also had replaced all (!) semicolons with commas. Quite a long of editing to do for me 😕
Hi Matt,
Thanks for the heads up on the $ interpolation feature – been using this for years in my other environments. However, I can report exactly the same issue as others have described; after publishing or reloading the $ is replaced by Concatenate in every instance!
I have the same issue and am on authoring version 3.22042.8
Hi Matthew, very cool feature! Would make coding quite a bit simpler and easier to read if it worked properly 😅.
Works perfect in Power Apps Studio. But the strings are invisible after publishing the app.
Im on authoring version 3.22041.27.
Hi Matthew. Have you tried this in a HTML label? What I’m finding is it ‘works’ in the studio, but when I play the app after publishing, the label defaults to the original placeholder text for a HTML label.
I not sure if this is a bug, but I found after I use $ string and close and reopen the app, all of my $ strings converted from ‘$”‘ to ‘concatenate’ and threw errors because now the syntax is wrong. Wondering if anyone come across this issue. I am on authoring version 3.22042.8
There was unfortunately a bug in the initial release of this feature that causes this behavior in some scenarios. It has been fixed in 3.22051 which will be available soon.
thanks for the update. Good to know
Used it for the first time and things didn’t go well. Opened the project the next day and it redid all the formulas. Below is an example of what happened, unfortunately I used the new format quite a bit and am in the process of changing them to the way I know works.
How I put it in: $”Hello{Char(10)}World”
What it changed too: ConcatenateHello{Char(10)}World”
I did check version and I’m on 3.22044.32, any recommendations?
Great article! Thanks for sharing.
How would I code a carriage return to place text on the next line?
Jeff,
You can use the CHAR function and the code Char(13).
Thank you!
Hi Matt
I have 3 columns in the sharepoint list, which I want to combine using a powerautomate flow.
In the flow, I am using Concat function as below with a separator
concat(triggerOutputs()?[‘body/Group1′],’, ‘,triggerOutputs()?[‘body/Group2′],’,’ ,triggerOutputs()?[‘body/Group3’])
Group1: Test 1, Test 2
Group2: empty column
Group3: Test3, Test4
The output is: Test 1, Test 2, ,Test3, Test4
I need to remove the extra separator if any of the columns are empty.
Thanks in advance
Shefi,
This question is off-topic. Please ask your question in the Power Automate forums:
https://powerusers.microsoft.com/t5/Microsoft-Power-Automate/ct-p/MPACommunity
Hello sir,
If I’m referencing several fields on a form and if the fields are not selected or populated, how would you make this $-String formula just leave those fields blank. Any advice?